C# 如何实现支付回调的重试通知机制
时间: 2023-07-27 11:29:55 浏览: 51
在 C# 中实现支付回调的重试通知机制的一般流程如下:
1. 接收到支付回调请求。
2. 处理支付回调请求,如果处理失败,则记录请求信息到数据库中,并设置重试次数为 0。
3. 启动计时器,根据重试次数和时间间隔进行通知。
4. 计时器触发后,从数据库中读取未成功处理的请求信息,进行重试通知。
5. 如果重试成功,则从数据库中删除请求信息;如果重试失败,则将重试次数加 1,并更新数据库中的请求信息。
6. 重复步骤 4 和 5,直到达到最大重试次数。
下面是一个简单的 C# 代码示例,实现了支付回调的重试通知机制:
```csharp
using System;
using System.Collections.Generic;
using System.Data.SqlClient;
using System.ServiceProcess;
using System.Threading;
namespace PaymentNotificationService
{
public partial class PaymentNotificationService : ServiceBase
{
private Timer retryTimer;
private const int MAX_RETRY_COUNT = 8;
private const int TIME_INTERVAL_1 = 4 * 60 * 1000; // 4 minutes
private const int TIME_INTERVAL_2 = 10 * 60 * 1000; // 10 minutes
private const int TIME_INTERVAL_3 = 1 * 60 * 60 * 1000; // 1 hour
private const int TIME_INTERVAL_4 = 2 * 60 * 60 * 1000; // 2 hours
private const int TIME_INTERVAL_5 = 6 * 60 * 60 * 1000; // 6 hours
private const int TIME_INTERVAL_6 = 15 * 60 * 60 * 1000; // 15 hours
public PaymentNotificationService()
{
InitializeComponent();
}
protected override void OnStart(string[] args)
{
// 启动计时器
retryTimer = new Timer(new TimerCallback(RetryNotification), null, 0, Timeout.Infinite);
}
protected override void OnStop()
{
// 停止计时器
retryTimer.Change(Timeout.Infinite, Timeout.Infinite);
}
private void RetryNotification(object state)
{
// 从数据库中读取未成功处理的请求信息
List<PaymentNotification> notifications = GetUnprocessedNotifications();
foreach (PaymentNotification notification in notifications)
{
if (notification.RetryCount < MAX_RETRY_COUNT)
{
// 根据重试次数设置时间间隔
int timeInterval = 0;
switch (notification.RetryCount)
{
case 0:
timeInterval = 0;
break;
case 1:
timeInterval = TIME_INTERVAL_1;
break;
case 2:
timeInterval = TIME_INTERVAL_2;
break;
case 3:
timeInterval = TIME_INTERVAL_3;
break;
case 4:
timeInterval = TIME_INTERVAL_4;
break;
case 5:
timeInterval = TIME_INTERVAL_5;
break;
case 6:
timeInterval = TIME_INTERVAL_6;
break;
default:
break;
}
// 进行重试通知
bool success = Notify(notification);
if (success)
{
// 重试成功,从数据库中删除请求信息
DeleteNotification(notification);
}
else
{
// 重试失败,更新数据库中的请求信息
UpdateNotification(notification);
}
// 进入下一次计时
retryTimer.Change(timeInterval, Timeout.Infinite);
}
else
{
// 达到最大重试次数,从数据库中删除请求信息
DeleteNotification(notification);
}
}
}
private List<PaymentNotification> GetUnprocessedNotifications()
{
// 从数据库中读取未成功处理的请求信息
List<PaymentNotification> notifications = new List<PaymentNotification>();
using (SqlConnection connection = new SqlConnection("your_connection_string"))
{
connection.Open();
SqlCommand command = new SqlCommand("SELECT * FROM PaymentNotifications WHERE RetryCount < @maxRetryCount", connection);
command.Parameters.AddWithValue("@maxRetryCount", MAX_RETRY_COUNT);
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
PaymentNotification notification = new PaymentNotification();
notification.Id = reader.GetInt32(0);
notification.OrderId = reader.GetString(1);
notification.Amount = reader.GetDecimal(2);
notification.RetryCount = reader.GetInt32(3);
notification.CreateTime = reader.GetDateTime(4);
notifications.Add(notification);
}
reader.Close();
}
return notifications;
}
private bool Notify(PaymentNotification notification)
{
// 实现通知的具体逻辑
// ...
return true;
}
private void UpdateNotification(PaymentNotification notification)
{
// 更新数据库中的请求信息
using (SqlConnection connection = new SqlConnection("your_connection_string"))
{
connection.Open();
SqlCommand command = new SqlCommand("UPDATE PaymentNotifications SET RetryCount = @retryCount WHERE Id = @id", connection);
command.Parameters.AddWithValue("@retryCount", notification.RetryCount + 1);
command.Parameters.AddWithValue("@id", notification.Id);
command.ExecuteNonQuery();
}
}
private void DeleteNotification(PaymentNotification notification)
{
// 从数据库中删除请求信息
using (SqlConnection connection = new SqlConnection("your_connection_string"))
{
connection.Open();
SqlCommand command = new SqlCommand("DELETE FROM PaymentNotifications WHERE Id = @id", connection);
command.Parameters.AddWithValue("@id", notification.Id);
command.ExecuteNonQuery();
}
}
}
public class PaymentNotification
{
public int Id { get; set; }
public string OrderId { get; set; }
public decimal Amount { get; set; }
public int RetryCount { get; set; }
public DateTime CreateTime { get; set; }
}
}
```
在上面的代码中,我们使用了一个 `Timer` 计时器来实现支付回调的重试通知机制。在 `OnStart` 方法中启动计时器,在 `OnStop` 方法中停止计时器。计时器在 `RetryNotification` 方法中触发,从数据库中读取未成功处理的请求信息,进行重试通知。在 `Notify` 方法中实现了具体的通知逻辑,您可以根据自己的需求进行修改。在 `UpdateNotification` 和 `DeleteNotification` 方法中分别实现了更新和删除请求信息的逻辑。同时,代码中的时间间隔参数也可以根据您的需求进行修改。
相关推荐
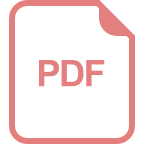
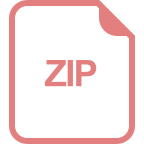














