模拟退火算法求解车辆路径问题代码
时间: 2023-09-06 11:08:09 浏览: 80
以下是一个使用模拟退火算法求解车辆路径问题的Python代码示例:
```python
import math
import random
# 城市坐标
CITIES = [(60, 200), (180, 200), (80, 180), (140, 180), (20, 160), (100, 160), (200, 160), (140, 140), (40, 120), (100, 120), (180, 100), (60, 80), (120, 80), (180, 60), (20, 40), (100, 40), (200, 40), (20, 20), (60, 20), (160, 20)]
# 计算两个城市之间的距离
def distance(city1, city2):
x1, y1 = city1
x2, y2 = city2
return math.sqrt((x1 - x2) ** 2 + (y1 - y2) ** 2)
# 计算路径总长度
def path_length(path):
total_length = 0.0
for i in range(len(path)):
city1 = path[i]
city2 = path[(i + 1) % len(path)]
total_length += distance(city1, city2)
return total_length
# 模拟退火算法求解车辆路径问题
def anneal():
# 初始化
temperature = 100000.0
cooling_rate = 0.003
current_path = CITIES[:]
best_path = CITIES[:]
current_length = path_length(current_path)
best_length = current_length
# 迭代
while temperature > 1:
# 生成新解
new_path = current_path[:]
index1 = random.randint(0, len(new_path) - 1)
index2 = random.randint(0, len(new_path) - 1)
new_path[index1], new_path[index2] = new_path[index2], new_path[index1]
new_length = path_length(new_path)
# 判断是否接受新解
delta = new_length - current_length
if delta < 0 or random.random() < math.exp(-delta / temperature):
current_path = new_path[:]
current_length = new_length
# 更新最优解
if current_length < best_length:
best_path = current_path[:]
best_length = current_length
# 降温
temperature *= 1 - cooling_rate
return best_path, best_length
# 测试
best_path, best_length = anneal()
print("最优路径:", best_path)
print("最优路径长度:", best_length)
```
在上述代码中,我们先定义了一个包含城市坐标的列表`CITIES`,然后定义了`distance()`函数用于计算两个城市之间的距离,以及`path_length()`函数用于计算路径总长度。接着我们定义了`anneal()`函数来实现模拟退火算法。
在`anneal()`函数中,我们首先初始化了一些参数,包括初始温度、冷却速率、当前路径、最优路径以及它们的长度。然后在一个循环中,不断生成新解并判断是否接受新解,同时更新最优解和降温。最后返回最优路径和最优路径长度。
最后在测试代码中调用`anneal()`函数并输出最优路径和最优路径长度。
阅读全文
相关推荐
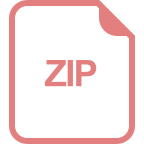

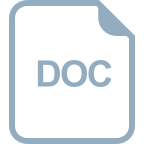
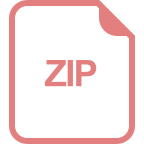
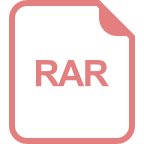
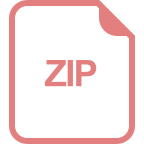
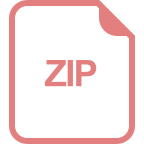
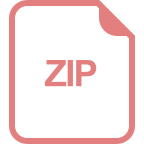
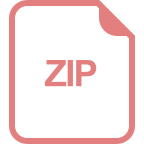
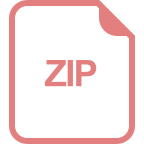
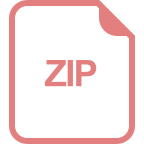
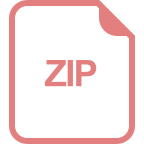
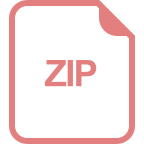
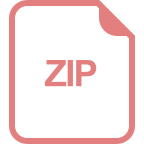
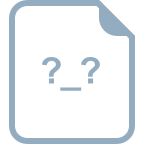
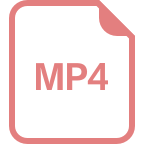