C++ 可以选择串口的打印函数例程
时间: 2024-09-08 18:01:04 浏览: 25
在C++中,串口通信通常涉及到硬件操作,因此需要使用操作系统提供的API或者特定库来处理串口。如果你正在使用Windows系统,可以考虑`SerialPort`类,它是Microsoft的`<serial>`库的一部分;对于Linux,通常使用POSIX API,如`termios.h`库。
以下是一个简单的例子,展示了如何在Windows上使用`#include <windows.h>`和`#include <winbase.h>`头文件通过`CreateFile`, `WriteFile`等函数发送数据到串口:
```cpp
#include <windows.h>
#include <winbase.h>
void print_to_serial(const char* port_name, const std::string& message) {
HANDLE hCom = CreateFile(port_name, GENERIC_READ | GENERIC_WRITE, 0, NULL,
OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if (hCom != INVALID_HANDLE_VALUE) {
DWORD bytes_written;
WriteFile(hCom, message.c_str(), message.length(), &bytes_written, NULL);
CloseHandle(hCom);
} else {
std::cerr << "Failed to open serial port." << std::endl;
}
}
```
如果你使用的是Linux,并且已经安装了`libserialport-dev`或其他相关的串口库,你可以使用`spoll()`函数配合`struct pollfd`结构体来实现异步读写:
```cpp
#include <sys/ioctl.h>
#include <fcntl.h>
#include <poll.h>
void print_to_serial(const char* port_name, const std::string& message) {
int fd = open(port_name, O_RDWR | O_NOCTTY | O_NDELAY); // 非阻塞打开
if (fd != -1) {
ioctl(fd, TIOCSBRK, 0); // 初始化
write(fd, message.c_str(), message.length()); // 写入数据
struct pollfd fds[1];
fds[0].fd = fd;
fds[0].events = POLLIN;
while (poll(fds, 1, 1000) > 0) { // 等待接收完成
if (fds[0].revents & POLLIN) break;
}
close(fd);
} else {
perror("Failed to open serial port.");
}
}
```
相关推荐
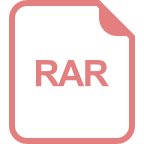
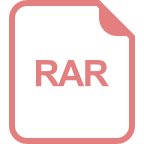
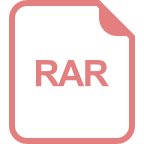














