能帮我编一个python三维可视化a星算法吗
时间: 2023-06-04 22:06:54 浏览: 163
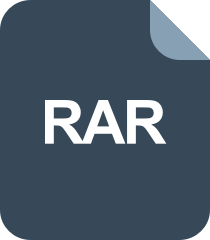
A星算法的代码

当然可以!以下是一个简单的 Python 三维可视化 A* 算法的示例(使用 matplotlib 和 mplot3d 库):
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 创建 3D 坐标系
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 构建地图
map_size = (10, 10, 10)
obstacle_centers = [(3,3,3), (2,2,2), (5,5,5), (7,7,7)]
obstacle_radii = [1, 1.5, 0.8, 1]
start = (0,0,0)
goal = (9,9,9)
# 计算启发函数 H
def heuristic(node):
return np.linalg.norm(np.array(node) - np.array(goal))
# 构建 A* 算法
def A_star(start, goal, obstacles):
open_set = set([start])
closed_set = set()
came_from = {}
g_score = {start: 0}
f_score = {start: heuristic(start)}
while open_set:
current = min(open_set, key=f_score.get)
if current == goal:
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.append(start)
path.reverse()
return path
open_set.remove(current)
closed_set.add(current)
for dx, dy, dz in [(1,0,0), (-1,0,0), (0,1,0), (0,-1,0), (0,0,1), (0,0,-1)]:
neighbor = (current[0]+dx, current[1]+dy, current[2]+dz)
if neighbor in closed_set or neighbor in obstacles:
continue
tentative_g_score = g_score[current] + np.linalg.norm(np.array(neighbor) - np.array(current))
if neighbor not in open_set or tentative_g_score < g_score[neighbor]:
came_from[neighbor] = current
g_score[neighbor] = tentative_g_score
f_score[neighbor] = tentative_g_score + heuristic(neighbor)
if neighbor not in open_set:
open_set.add(neighbor)
return None
# 绘制地图和路径
x, y, z = np.indices(map_size)
x = x.ravel()
y = y.ravel()
z = z.ravel()
ax.scatter(x, y, z, color='gray', alpha=0.1, s=10)
for center, radius in zip(obstacle_centers, obstacle_radii):
u, v = np.mgrid[0:2*np.pi:20j, 0:np.pi:10j]
x = radius * np.cos(u) * np.sin(v) + center[0]
y = radius * np.sin(u) * np.sin(v) + center[1]
z = radius * np.cos(v) + center[2]
ax.plot_wireframe(x, y, z, color="gray", alpha=0.5)
start_node = ax.scatter(start[0], start[1], start[2], color='green', marker='o')
goal_node = ax.scatter(goal[0], goal[1], goal[2], color='red', marker='o')
path = A_star(start, goal, obstacle_centers)
if path:
path_x, path_y, path_z = zip(*path)
ax.plot(path_x, path_y, path_z, color='blue', linewidth=2)
plt.show()
```
运行以上代码,即可得到一个类似以下的三维可视化效果:

阅读全文
相关推荐
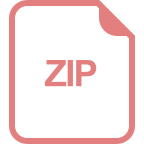
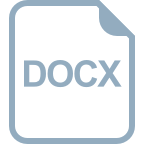
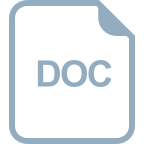
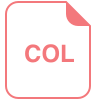
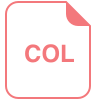
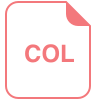
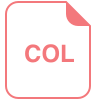
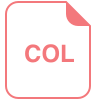
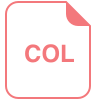
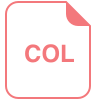
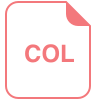
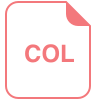
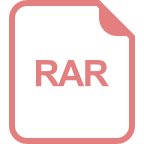