使用OpenGL 实现 代码弹幕” 200312225 OpenGL”从上到下,循环反复
时间: 2024-06-10 10:10:59 浏览: 11
这是一个简单的使用OpenGL实现弹幕的代码示例,它将在OpenGL窗口中从上到下显示“200312225 OpenGL”,然后循环反复。
```c++
#include <iostream>
#include <string>
#include <GL/glut.h>
using namespace std;
int windowWidth = 800;
int windowHeight = 600;
int xPos = 0;
int yPos = windowHeight;
string text = "200312225 OpenGL";
void display() {
// Clear the buffer
glClear(GL_COLOR_BUFFER_BIT);
// Set the text color
glColor3f(1.0, 1.0, 1.0);
// Set the text position
glRasterPos2f(xPos, yPos);
// Draw the text
for (int i = 0; i < text.length(); i++) {
glutBitmapCharacter(GLUT_BITMAP_TIMES_ROMAN_24, text[i]);
}
// Swap buffers to show the frame
glutSwapBuffers();
}
void timer(int value) {
// Move the text upwards
yPos -= 5;
// If the text has reached the bottom of the window, reset its position
if (yPos <= -25) {
yPos = windowHeight;
}
// Redraw the scene
glutPostRedisplay();
// Set the timer to call itself again after 50 milliseconds
glutTimerFunc(50, timer, 0);
}
int main(int argc, char** argv) {
// Initialize GLUT
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB);
glutInitWindowSize(windowWidth, windowHeight);
glutCreateWindow("OpenGL Danmaku");
// Set the clear color to black
glClearColor(0.0, 0.0, 0.0, 1.0);
// Set the projection matrix
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0, windowWidth, 0, windowHeight);
// Set the modelview matrix
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
// Set the timer to call the timer function after 50 milliseconds
glutTimerFunc(50, timer, 0);
// Start the GLUT main loop
glutMainLoop();
return 0;
}
```
在这个示例中,我们使用了GLUT库来创建OpenGL窗口并处理事件。在主函数中,我们首先初始化GLUT,然后设置窗口的大小和标题。接下来,我们设置了清除颜色为黑色,并将投影矩阵和模型视图矩阵设置为2D正交矩阵,以便在窗口中绘制文本。
在显示函数中,我们首先清除了颜色缓冲区,然后设置了文本颜色为白色,并将文本位置设置为当前的xPos和yPos。我们使用了GLUT提供的glRasterPos2f函数来设置文本位置,这个函数接受两个参数,分别是x和y坐标。接下来,我们使用一个循环来遍历文本中的所有字符,并使用GLUT提供的glutBitmapCharacter函数将每个字符绘制到屏幕上。
在计时器函数中,我们将yPos的值减去5,以向上移动文本。如果文本已经到达了窗口的底部(yPos小于等于-25),我们将它的位置重置为窗口的高度。最后,我们使用glutPostRedisplay函数重新绘制屏幕,并使用glutTimerFunc函数在50毫秒后再次调用计时器函数,以便实现循环弹幕效果。
请注意,此示例中使用的GLUT库已经过时,您可以使用更现代的OpenGL框架,例如GLFW或SDL,来创建窗口并处理事件。
相关推荐














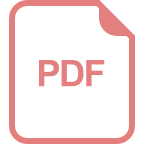