代码
时间: 2023-07-02 08:22:43 浏览: 103
好的,这是一个比较复杂的系统,需要涉及到多个模块和技术。以下是一个大致的代码框架:
1. 题库模块:包括题目的存储、随机抽取、答案校验等功能。
```
class Question:
def __init__(self, prompt, options, answer):
self.prompt = prompt
self.options = options
self.answer = answer
class QuestionBank:
def __init__(self):
self.questions = [] # 存储题目的列表
def add_question(self, question):
self.questions.append(question)
def get_random_questions(self, num):
return random.sample(self.questions, num)
def check_answer(self, question, answer):
return question.answer == answer
```
2. 考试模块:包括考试时间、答题过程管理、终止考试等功能。
```
class Exam:
def __init__(self, question_bank, time_limit_in_minutes):
self.question_bank = question_bank
self.time_limit = time_limit_in_minutes * 60
self.start_time = None
self.end_time = None
self.questions = None
self.answers = None
self.score = None
def start_exam(self):
self.questions = self.question_bank.get_random_questions(100)
self.answers = [None] * len(self.questions)
self.start_time = time.time()
self.end_time = self.start_time + self.time_limit
def answer_question(self, index, answer):
self.answers[index] = answer
def submit_exam(self):
self.end_time = time.time()
self.score = 0
num_answered = 0
num_correct = 0
num_wrong = 0
num_unanswered = 0
for i in range(len(self.questions)):
if self.answers[i] is not None:
num_answered += 1
if self.question_bank.check_answer(self.questions[i], self.answers[i]):
num_correct += 1
self.score += 1
else:
num_wrong += 1
self.score -= 11
if num_wrong >= 11:
self.end_time = time.time()
break
else:
num_unanswered += 1
return {
'score': self.score,
'num_correct': num_correct,
'num_wrong': num_wrong,
'num_unanswered': num_unanswered,
'time_taken': self.end_time - self.start_time,
'num_answered': num_answered
}
```
3. 用户界面模块:包括用户输入、界面显示、交互等功能。
```
class UserInterface:
def __init__(self, exam):
self.exam = exam
def start(self):
self.exam.start_exam()
while True:
self.show_question()
answer = self.get_answer()
if answer == 'submit':
result = self.exam.submit_exam()
self.show_result(result)
break
else:
self.exam.answer_question(self.current_question_index, answer)
self.current_question_index += 1
if self.current_question_index >= len(self.exam.questions):
self.current_question_index = 0
def show_question(self):
question = self.exam.questions[self.current_question_index]
print(question.prompt)
for i, option in enumerate(question.options):
print(f'{i+1}. {option}')
def get_answer(self):
while True:
answer = input('请输入答案,或输入"submit"交卷:')
if answer.isdigit() and int(answer) in range(1, 5):
return int(answer) - 1
elif answer.lower() == 'submit':
return 'submit'
else:
print('答案不合法,请重新输入')
def show_result(self, result):
print('考试结束,统计结果如下:')
print(f'总分:{result["score"]}')
print(f'答对题数:{result["num_correct"]}')
print(f'答错题数:{result["num_wrong"]}')
print(f'未答题数:{result["num_unanswered"]}')
print(f'用时:{result["time_taken"] / 60} 分钟')
```
这里只是提供了一个大致的框架,实际上还需要考虑很多细节,比如如何读取题库文件、如何处理用户输入错误、如何将结果保存到数据库等等。如果您有具体的问题或需要更详细的代码,可以再告诉我。
阅读全文
相关推荐



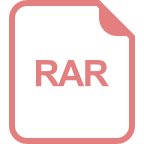




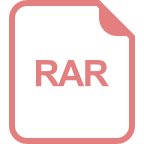