java如何在excel表格中的第5行后面添加一行并在第2列和第3列写入数据,要求不能覆盖第6行以及后面所有的数据,请写出详细的代码
时间: 2024-04-12 10:32:18 浏览: 219
要在Excel表格中的第5行后面添加一行,并在第2列和第3列写入数据,同时确保不覆盖第6行及其后面的数据,可以使用Apache POI库来实现。下面是一个示例代码:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class ExcelWriter {
public static void main(String[] args) {
String filePath = "path/to/excel.xlsx"; // Excel文件路径
int startRow = 5; // 开始行数
int insertRow = startRow + 1; // 插入行数
try (FileInputStream inputStream = new FileInputStream(filePath);
Workbook workbook = new XSSFWorkbook(inputStream)) {
Sheet sheet = workbook.getSheetAt(0); // 获取第一个工作表
// 获取第5行及其后面的行数
int totalRows = sheet.getLastRowNum() + 1;
int remainingRows = totalRows - startRow;
// 向下移动行
sheet.shiftRows(insertRow, totalRows, 1, true, false);
Row newRow = sheet.createRow(insertRow); // 创建新行
// 在第2列和第3列写入数据
String[] data = {"Data1", "Data2"};
for (int i = 0; i < data.length; i++) {
Cell cell = newRow.createCell(i + 1);
cell.setCellValue(data[i]);
}
try (FileOutputStream outputStream = new FileOutputStream(filePath)) {
workbook.write(outputStream);
}
System.out.println("数据已成功添加到Excel文件!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上代码会在Excel表格中的第5行后面插入一行,并在第2列和第3列写入指定的数据。插入新行后,原有的第6行及其后面的数据会自动下移一行,不会被覆盖。确保在运行代码之前已经添加了Apache POI库的依赖。
阅读全文
相关推荐





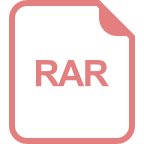







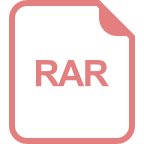

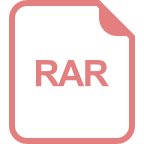