edge浏览器发送delete请求
时间: 2023-09-15 14:17:49 浏览: 126
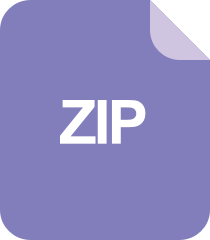
卸载Edge浏览器

要发送 DELETE 请求,您可以使用 JavaScript 的 Fetch API 或 XMLHttpRequest API。以下是一个使用 Fetch API 发送 DELETE 请求的示例:
```javascript
fetch('your-url', {
method: 'DELETE',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({data: 'your-data'})
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error('There was a problem with the fetch operation:', error);
});
```
在上面的代码中,您需要将 `your-url` 替换为您要发送 DELETE 请求的 URL,将 `your-data` 替换为您要在请求主体中发送的数据。请注意,此示例使用 JSON 数据,因此设置了 `Content-Type` 请求标头。如果您使用不同的数据类型,请相应地更改标头。
如果您使用 XMLHttpRequest API 发送 DELETE 请求,则可以使用以下代码:
```javascript
const xhr = new XMLHttpRequest();
xhr.open('DELETE', 'your-url');
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.onload = function() {
if (xhr.status === 200) {
const data = JSON.parse(xhr.responseText);
console.log(data);
} else {
console.error('There was a problem with the request.');
}
};
xhr.send(JSON.stringify({data: 'your-data'}));
```
同样,您需要将 `your-url` 替换为您要发送 DELETE 请求的 URL,将 `your-data` 替换为您要在请求主体中发送的数据。此示例也使用 JSON 数据。如果您使用不同的数据类型,请相应地更改标头和发送的数据。
阅读全文
相关推荐
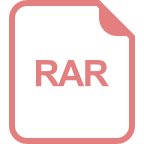
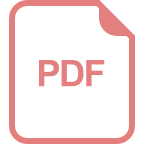
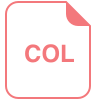

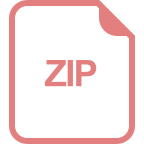
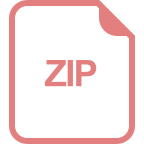
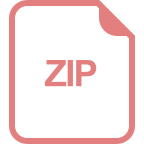