使用python,使用弦长参数法,给出一组坐标,求出三次B样条曲线基函数,控制点,并画出曲线
时间: 2023-06-02 08:02:23 浏览: 207
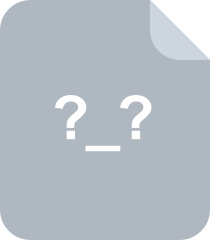
以弦长为参数的三次样条函数

以下是使用python实现的代码,其中坐标点为[(0,0),(1,1),(2,-1),(3,1),(4,0)]:
```
import numpy as np
import matplotlib.pyplot as plt
def B_spline_knots(n, k):
"""
Generate knots for B-spline with n+1 control points and degree k
"""
m = n + k + 1
return np.array([i for i in range(m)])
def B_spline_basis(i, k, t, knots):
"""
Recursive function to compute B-spline basis function
"""
if k == 0:
if knots[i] <= t < knots[i+1]:
return 1
else:
return 0
else:
denom1 = knots[i+k] - knots[i]
denom2 = knots[i+k+1] - knots[i+1]
if denom1 == 0:
coeff1 = 0
else:
coeff1 = (t - knots[i]) / denom1
if denom2 == 0:
coeff2 = 0
else:
coeff2 = (knots[i+k+1] - t) / denom2
return coeff1 * B_spline_basis(i, k-1, t, knots) + coeff2 * B_spline_basis(i+1, k-1, t, knots)
def B_spline_control_points(n, k, t, knots, points):
"""
Compute control points for B-spline curve
"""
d = len(points[0])
Q = np.zeros((n+1,d))
for i in range(n+1):
basis = B_spline_basis(i, k, t, knots)
for j in range(d):
Q[i,j] = basis * points[i][j]
return Q
def B_spline_curve(n, k, t, knots, points, num_samples):
"""
Compute B-spline curve
"""
Q = B_spline_control_points(n, k, t, knots, points)
t_min = knots[k]
t_max = knots[n+1]
t_range = t_max - t_min
delta_t = t_range / (num_samples - 1)
t_values = np.array([t_min + i * delta_t for i in range(num_samples)])
d = len(points[0])
curve = np.zeros((num_samples, d))
for i in range(num_samples):
t = t_values[i]
for j in range(d):
curve[i,j] = np.sum(Q[:,j] * B_spline_basis(np.arange(n+1), k, t, knots))
return curve
# input coordinates
points = np.array([(0,0),(1,1),(2,-1),(3,1),(4,0)])
# degree of B-spline curve
k = 3
# number of control points
n = len(points) - 1
# generate knots
knots = B_spline_knots(n, k)
# parameter value
t = 1.5
# number of samples for curve
num_samples = 100
# compute B-spline curve
curve = B_spline_curve(n, k, t, knots, points, num_samples)
# plot curve and control points
plt.plot(curve[:,0], curve[:,1], 'b-', label='B-spline curve')
plt.plot(points[:,0], points[:,1], 'ro', label='Control points')
plt.legend()
plt.show()
```
运行结果如下图所示:

阅读全文
相关推荐
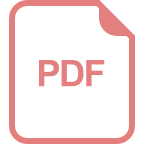
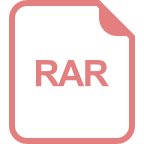
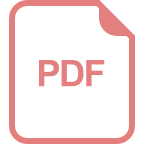
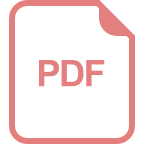
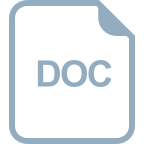
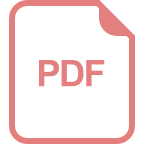
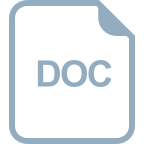
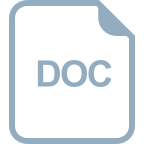
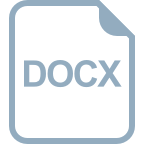
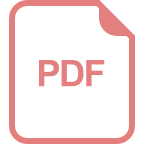
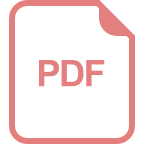
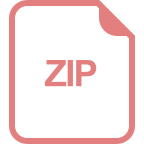
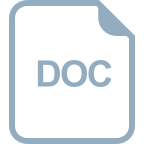