这段代码是什么意思public class CalendarBean { String day[]; int year=2022,month=0; public void setYear(int year) { this.year=year; } public int getYear() { return year; } public void setMonth(int month) { this.month=month; } public int getMonth() { return month; } public String[] getCalendar() { String a[]=new String[42]; Calendar date=Calendar.getInstance(); date.set(year,month-1,1); int week=date.get(Calendar.DAY_OF_WEEK)-1; int day=0; //判断大月份 if(month==1||month==3||month==5||month==7 ||month==8||month==10||month==12) { day=31; } //判断小月 if(month==4||month==6||month==9||month==11) { day=30; } //判断平年与闰年 if(month==2) { if(((year%4==0)&&(year%100!=0))||(year%400==0)) { day=29; } else { day=28; } } for(int i=week,n=1;i<week+day;i++) { a[i]=String.valueOf(n) ; n++; } return a; } }
时间: 2024-02-10 11:35:10 浏览: 69
这段代码定义了一个名为 `CalendarBean` 的 Java 类,该类包含了一些方法和属性,其作用是生成一个指定年份和月份的日历。其中,`day` 属性是一个字符串数组,存储日历中每一天的日期;`year` 和 `month` 属性分别表示所生成日历的年份和月份。类中包含了几个方法,如 `setYear`、`getYear`、`setMonth`、`getMonth` 等方法,用于设置和获取年份和月份。最重要的方法是 `getCalendar`,它根据所设定的年份和月份,生成一个包含 42 个元素的字符串数组 `a`,表示该月份的日历。具体的生成方式是,首先通过 `Calendar` 类获取该月份的第一天是星期几,然后根据月份的天数判断每一天的日期,最后将每一天的日期存入 `a` 数组中。
相关问题
MyDate属性包括:year,month,day
在`MyDate`类中,如果我们按照您的描述,它应该是一个代表日期的对象,包含了年份(year)、月份(month)和日期(day)三个基本属性。下面是一个简单的`MyDate`类的示例:
```java
public class MyDate {
private int year;
private int month;
private int day;
// 构造函数
public MyDate(int year, int month, int day) {
if (year < 0 || month < 1 || month > 12 || day < 1) {
throw new IllegalArgumentException("Invalid date");
}
this.year = year;
this.month = month;
this.day = day;
}
// Getter methods for the properties
public int getYear() {
return year;
}
public int getMonth() {
return month;
}
public int getDay() {
return day;
}
// Setter methods to modify the properties
public void setYear(int year) {
this.year = year;
}
public void setMonth(int month) {
this.month = month;
}
public void setDay(int day) {
this.day = day;
}
// Overriden equals(), hashCode() and toString() methods for better object handling
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
MyDate other = (MyDate) obj;
return year == other.year && month == other.month && day == other.day;
}
@Override
public int hashCode() {
return Objects.hash(year, month, day);
}
@Override
public String toString() {
return String.format("%d-%02d-%02d", year, month, day);
}
}
```
在这个类中,我们设置了构造函数来验证输入的日期是否有效,并提供了getter和setter方法来操作各个属性。同时,还覆盖了`equals()`、`hashCode()`和`toString()`方法,以支持日期比较和其他常见操作。
在实验三中曾经实现过日期类MyDate,它包含一个含参数的构造方法MyDate(intyear,intmonth,intday)、以及三个相应的设置方法setXXX(intarg)。1.现编写一个自定义的异常InvalidDateException。当构造方法或设置方法接受的参数不符合实际要求时,抛出一个这个异常的实例。2.在main方法中分别包含以下代码,捕获并打印异常信息:MyDated1=newMyDate(-1,13,32);MyDated2=newMyDate();d2.setDay(100);
以下是实现代码:
```java
class InvalidDateException extends Exception {
public InvalidDateException(String message) {
super(message);
}
}
class MyDate {
private int year;
private int month;
private int day;
public MyDate(int year, int month, int day) throws InvalidDateException {
if (year <= 0 || month <= 0 || month > 12 || day <= 0 || day > 31) {
throw new InvalidDateException("Invalid date");
}
this.year = year;
this.month = month;
this.day = day;
}
public MyDate() {
this.year = 1;
this.month = 1;
this.day = 1;
}
public void setYear(int year) throws InvalidDateException {
if (year <= 0) {
throw new InvalidDateException("Invalid year");
}
this.year = year;
}
public void setMonth(int month) throws InvalidDateException {
if (month <= 0 || month > 12) {
throw new InvalidDateException("Invalid month");
}
this.month = month;
}
public void setDay(int day) throws InvalidDateException {
if (day <= 0 || day > 31) {
throw new InvalidDateException("Invalid day");
}
this.day = day;
}
}
public class Main {
public static void main(String[] args) {
try {
MyDate d1 = new MyDate(-1, 13, 32);
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
MyDate d2 = new MyDate();
try {
d2.setDay(100);
} catch (InvalidDateException e) {
System.out.println(e.getMessage());
}
}
}
```
输出结果为:
```
Invalid date
Invalid day
```
阅读全文
相关推荐
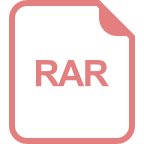
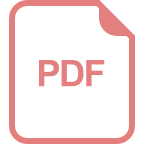
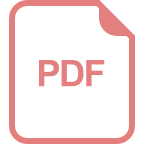













