如何使用Elasticsearch的Java API连接Elasticsearch集群?
时间: 2023-12-20 08:29:14 浏览: 38
要使用Elasticsearch的Java API连接Elasticsearch集群,需要添加以下三个依赖:org.elasticsearch.client.elasticsearch-rest-high-level-client、org.elasticsearch.client:elasticsearch-rest-client、org.elasticsearch:elasticsearch。这些依赖可以通过Maven或Gradle添加到项目中。接下来,可以使用Elasticsearch的Java API编写代码来连接Elasticsearch集群。具体步骤如下:
1. 创建RestHighLevelClient对象,该对象是与Elasticsearch集群进行通信的主要入口点。
2. 创建IndexRequest对象,该对象表示要索引的文档。
3. 使用IndexRequest对象设置索引名称、文档类型和文档ID等信息。
4. 使用IndexRequest对象设置要索引的文档内容。
5. 使用RestHighLevelClient对象执行IndexRequest对象,将文档索引到Elasticsearch集群中。
下面是一个示例代码,用于将文档索引到Elasticsearch集群中:
```java
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.action.index.IndexResponse;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.common.xcontent.XContentType;
import org.elasticsearch.index.IndexNotFoundException;
import org.elasticsearch.rest.RestStatus;
import java.io.IOException;
public class ElasticsearchExample {
private RestHighLevelClient client;
public ElasticsearchExample(RestHighLevelClient client) {
this.client = client;
}
public void indexDocument(String index, String type, String id, String json) throws IOException {
IndexRequest request = new IndexRequest(index, type, id);
request.source(json, XContentType.JSON);
IndexResponse response = client.index(request, RequestOptions.DEFAULT);
if (response.status() == RestStatus.CREATED) {
System.out.println("Document indexed successfully");
} else {
System.out.println("Failed to index document");
}
}
public static void main(String[] args) throws IOException {
RestHighLevelClient client = new RestHighLevelClient(/* Elasticsearch连接配置 */);
ElasticsearchExample example = new ElasticsearchExample(client);
example.indexDocument("my_index", "my_type", "1", "{\"name\":\"John Doe\",\"age\":25}");
client.close();
}
}
相关推荐
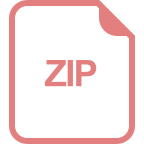
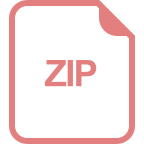
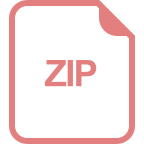











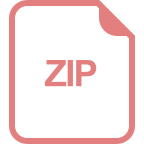
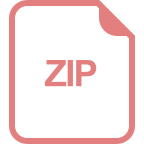
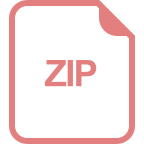
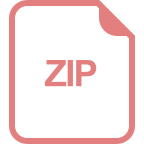