qt C++ UI创建一个frame,名字为frame_videoPlayer 用于视频播放器区域,创建一个视频播放器 , 文件从本地获取选择
时间: 2024-09-14 18:06:22 浏览: 47
在Qt C++中创建一个名为`frame_videoPlayer`的框架作为视频播放器区域,你需要按照以下步骤操作:
1. **包含必要的头文件**:
```cpp
#include <QApplication>
#include <QWidget>
#include <QFrame>
#include <QFileDialog>
#include <QMediaPlayer>
#include <QPushButton>
```
这里包含了基本UI元素、媒体播放器以及文件对话框。
2. **定义主窗口类** (假设名为VideoPlayerApp),并继承自QMainWindow:
```cpp
class VideoPlayerApp : public QMainWindow {
Q_OBJECT
public:
VideoPlayerApp(QWidget *parent = nullptr);
~VideoPlayerApp();
private slots:
void openVideoFile();
private:
QWidget *centralWidget;
QFrame *frame_videoPlayer;
QPushButton *openButton;
QMediaPlayer *mediaPlayer;
};
```
3. **构造函数和析构函数**:
```cpp
VideoPlayerApp::VideoPlayerApp(QWidget *parent) : QMainWindow(parent) {
// 初始化UI组件
centralWidget = new QWidget(this);
setCentralWidget(centralWidget);
frame_videoPlayer = new QFrame(frame_videoPlayer);
// 设置样式等属性
...
openButton = new QPushButton("打开", this);
openButton->setFixedSize(100, 50);
connect(openButton, &QPushButton::clicked, this, &VideoPlayerApp::openVideoFile);
openButton->move(QPoint(//位置));
// 添加到布局
QVBoxLayout *layout = new QVBoxLayout(centralWidget);
layout->addWidget(frame_videoPlayer);
layout->addWidget(openButton);
}
VideoPlayerApp::~VideoPlayerApp() {}
```
4. **实现打开文件槽函数** `openVideoFile()`:
```cpp
void VideoPlayerApp::openVideoFile() {
QString filePath = QFileDialog::getOpenFileName(this, "选择视频文件", "", tr("Video Files (*.mp4 *.avi);;All Files (*)"));
if (!filePath.isEmpty()) {
mediaPlayer->setMedia(QMediaContent(QUrl(filePath)));
mediaPlayer->play(); // 播放视频
}
}
```
这会在点击按钮时弹出文件选择框,用户可以选择视频文件后开始播放。
注意:别忘了在`main.cpp`中创建`VideoPlayerApp`实例,并设置窗口大小和显示等其他细节。此外,记得处理可能出现的错误,比如播放失败的情况。
阅读全文
相关推荐
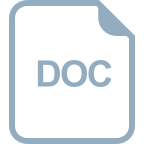
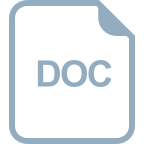
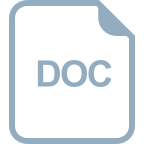
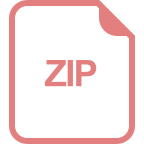
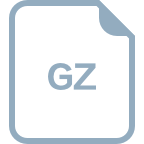
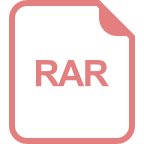
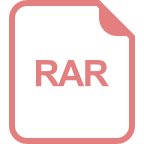
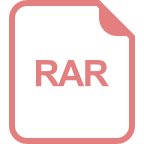
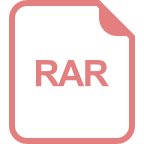
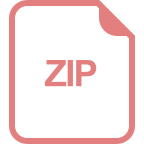
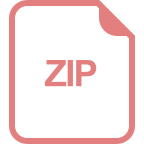
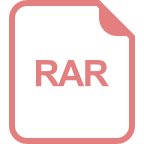
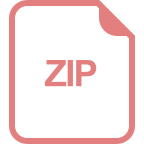
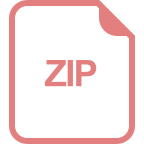
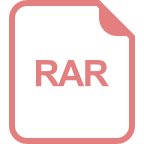
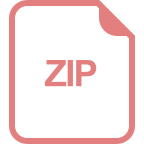
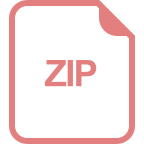
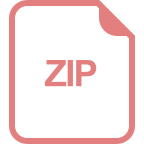