python编程实现调用aes对称加密算法对一段文本加解密
时间: 2024-11-25 22:31:10 浏览: 11
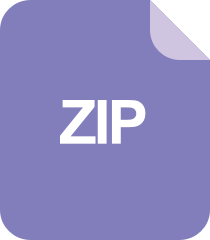
python之AES加密解密源码

在Python中,我们可以使用第三方库`pycryptodome`来实现AES对称加密。下面是一个简单的例子,展示如何对文本进行加密和解密:
首先,你需要安装`pycryptodome`库,可以使用pip安装:
```bash
pip install pycryptodome
```
然后,你可以按照以下步骤操作:
1. 导入必要的模块:
```python
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad, unpad
from base64 import b64encode, b64decode
```
2. 选择一个固定长度的密钥(16字节通常用于AES-128),并创建一个AES cipher对象:
```python
key = b'Sixteen byte key here'
cipher = AES.new(key, AES.MODE_ECB)
```
注意,ECB模式主要用于教学示例,实际应用中推荐使用CBC等更安全的模式。
3. 加密函数:
```python
def encrypt(text, key):
plaintext = pad(text.encode(), AES.block_size) # 对明文进行填充,保证块大小
ciphertext = cipher.encrypt(plaintext)
return b64encode(ciphertext).decode() # 使用Base64编码便于存储
# 示例:加密文本 "Hello, world!"
encrypted_text = encrypt("Hello, world!", key)
```
4. 解密函数:
```python
def decrypt(encrypted_text, key):
encoded_ciphertext = b64decode(encrypted_text)
decrypted_plaintext = unpad(cipher.decrypt(encoded_ciphertext), AES.block_size)
return decrypted_plaintext.decode()
# 示例:解密加密后的文本
decrypted_text = decrypt(encrypted_text, key)
print(f"Decrypted text: {decrypted_text}")
```
阅读全文
相关推荐
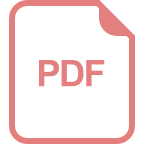
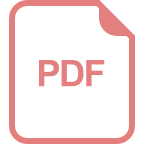

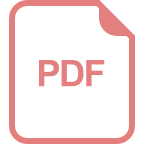
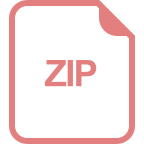
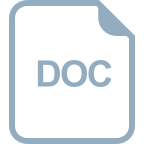
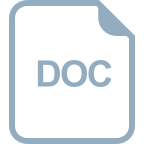
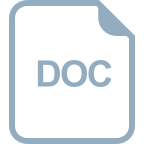
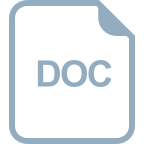
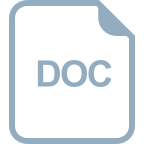
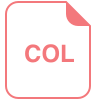
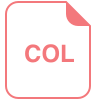




