python脚本实现GFF3转GTF
时间: 2024-08-29 20:00:47 浏览: 132
GFF3 (General Feature Format version 3) 和 GTF (Gene Transfer Format) 都是生物信息学中用于描述基因组特征的文件格式。GFF3通常包含更详细的注释信息,而GTF则简化了一些字段并按照特定的顺序组织。
要在Python中编写一个脚本来将GFF3转换为GTF,你可以利用pandas库处理CSV-like数据,并编写一些规则来格式化转换。以下是一个简单的示例,假设你有一个名为`input.gff3`的GFF3文件:
```python
import pandas as pd
def gff3_to_gtf(input_file, output_file):
# 读取GFF3文件
df = pd.read_csv(input_file, sep='\t', comment='#', header=None,
names=['seqid', 'source', 'type', 'start', 'end', 'score', 'strand',
'phase', 'attributes'])
# 简化和格式化GTF
df['attribute_dict'] = df.attributes.str.split(';').apply(dict)
df = df[['seqid', 'source', 'type', 'start', 'end', 'score', 'strand', 'phase']]
df['attributes'] = df.attribute_dict.apply(lambda x: ';'.join(f'{k} "{v}"' for k, v in x.items()))
# 转换列名和添加缺失的列
df.columns = ['chr', 'transcript_id', 'gene_name', 'start', 'end', 'score', 'strand', '.']
df = df[['chr', 'transcript_id', 'gene_name', 'start', 'end', 'score', 'strand', '.', 'phase', 'attributes']]
# 写入GTF文件
df.to_csv(output_file, sep='\t', index=False)
# 使用函数
gff3_to_gtf('input.gff3', 'output.gtf')
```
注意:这个例子假设GFF3文件的基本结构和GTF的预期字段相对应。实际操作中,如果GFF3文件的结构复杂,可能需要调整解析步骤。此外,GTF有一些特定的要求,如某些列的值可能需要特殊处理。
阅读全文
相关推荐
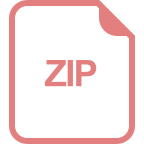
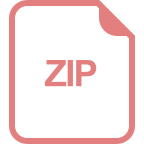
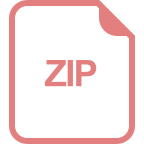
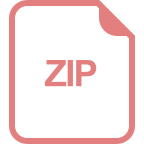
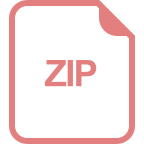
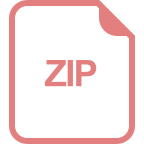
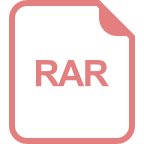
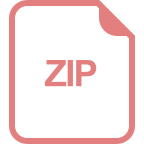
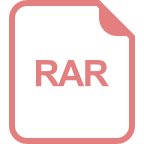
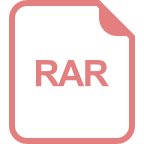
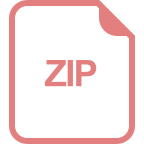
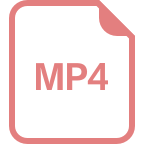
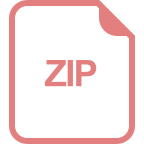