/proc/<pid>/stack文件的作用
时间: 2023-08-07 21:03:31 浏览: 143
/proc/<pid>/stack文件是Linux系统中的一个特殊文件,用于查看进程的堆栈信息。每个运行中的进程都有一个对应的/proc/<pid>/stack文件,其中的内容记录了该进程当前的函数调用栈信息。
通过读取/proc/<pid>/stack文件,你可以了解进程当前所执行的函数调用路径,即函数调用栈。这对于调试和分析进程的行为和性能问题非常有用。
/proc/<pid>/stack文件的内容通常以十六进制显示,每一行表示一个函数调用栈帧。每个栈帧包含了函数的返回地址和一些其他与函数调用相关的信息。
注意,/proc/<pid>/stack文件只显示进程当前的函数调用栈,因此在不同时间读取该文件可能会得到不同的结果。如果需要获取完整的函数调用栈信息,可以考虑使用其他调试工具,如gdb。
阅读全文
相关推荐
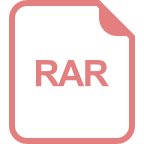
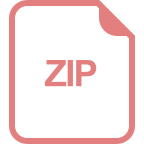
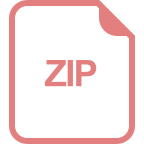
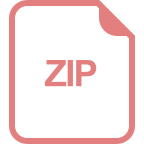
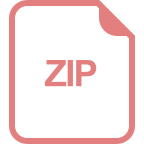