用c语言完成count函 数。函数功能:统计循环单 链表中值为n的数据的个数。
时间: 2024-09-21 10:13:16 浏览: 33
在C语言中,你可以定义一个名为`countNodes`的函数来完成这个任务。假设我们已经有了一个结构体`ListNode`表示链表节点,其中包含一个整数值`val`和指向下一个节点的指针`next`。以下是函数的基本实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val; // 节点值
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
// 函数声明
int countNodes(ListNode* head, int n);
// 定义链表节点的创建和插入操作(这里仅作示例,实际项目中可能已存在)
ListNode* createNode(int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->val = value;
newNode->next = NULL;
return newNode;
}
// 主函数演示如何使用countNodes
void testCountNodes() {
// 创建链表并添加一些节点
ListNode* list = createNode(1);
list->next = createNode(2);
list->next->next = createNode(3);
list->next->next->next = createNode(n); // 其中n是你想要计数的目标值
int result = countNodes(list, n);
printf("The number of nodes with value %d is: %d\n", n, result);
}
// 实现countNodes函数
int countNodes(ListNode* head, int n) {
int count = 0;
ListNode* current = head;
while (current != NULL) {
if (current->val == n) {
count++;
}
current = current->next;
}
return count;
}
```
在这个例子中,`countNodes`函数遍历整个链表,每当遇到值等于`n`的节点就增加计数器`count`。当你想统计特定值在链表中出现的次数时,只需调用`countNodes(head, n)`即可。
相关推荐
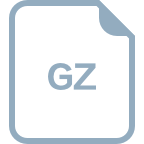
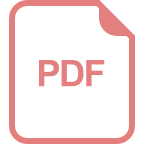
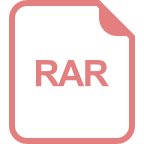

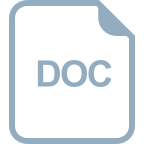

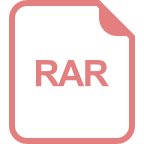
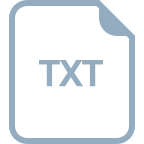
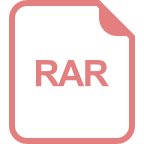
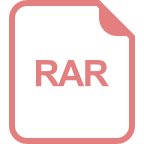
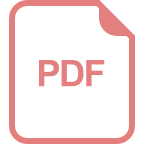
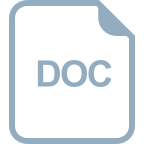
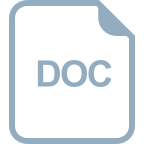
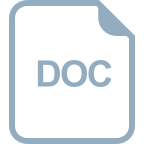
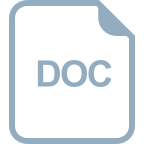
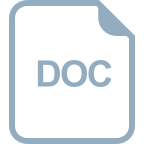
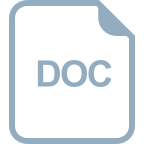