c语言双向链表通讯录代码
时间: 2023-12-25 18:29:34 浏览: 102
以下是一个使用C语言实现的双向链表通讯录代码的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义通讯录节点结构体
typedef struct Contact {
char name[50];
char phone[20];
struct Contact* prev;
struct Contact* next;
} Contact;
// 全局变量,指向通讯录头节点
Contact* head = NULL;
// 创建新的通讯录节点
Contact* createContact(char name[], char phone[]) {
Contact* newContact = (Contact*)malloc(sizeof(Contact));
strcpy(newContact->name, name);
strcpy(newContact->phone, phone);
newContact->prev = NULL;
newContact->next = NULL;
return newContact;
}
// 在通讯录末尾添加新的联系人
void addContact(char name[], char phone[]) {
Contact* newContact = createContact(name, phone);
if (head == NULL) {
head = newContact;
} else {
Contact* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newContact;
newContact->prev = current;
}
}
// 显示通讯录中的所有联系人
void displayContacts() {
Contact* current = head;
if (current == NULL) {
printf("通讯录为空。\n");
} else {
printf("通讯录中的联系人:\n");
while (current != NULL) {
printf("姓名:%s,电话:%s\n", current->name, current->phone);
current = current->next;
}
}
}
// 根据姓名查找联系人
void searchContact(char name[]) {
Contact* current = head;
while (current != NULL) {
if (strcmp(current->name, name) == 0) {
printf("姓名:%s,电话:%s\n", current->name, current->phone);
return;
}
current = current->next;
}
printf("未找到该联系人。\n");
}
// 删除指定姓名的联系人
void deleteContact(char name[]) {
Contact* current = head;
while (current != NULL) {
if (strcmp(current->name, name) == 0) {
if (current->prev == NULL) {
head = current->next;
if (head != NULL) {
head->prev = NULL;
}
} else {
current->prev->next = current->next;
if (current->next != NULL) {
current->next->prev = current->prev;
}
}
free(current);
printf("已删除联系人:%s\n", name);
return;
}
current = current->next;
}
printf("未找到该联系人。\n");
}
// 释放通讯录内存
void freeContacts() {
Contact* current = head;
while (current != NULL) {
Contact* temp = current;
current = current->next;
free(temp);
}
head = NULL;
}
int main() {
// 添加示例联系人
addContact("张三", "123456789");
addContact("李四", "987654321");
// 显示通讯录
displayContacts();
// 查找联系人
searchContact("张三");
// 删除联系人
deleteContact("李四");
// 显示通讯录
displayContacts();
// 释放通讯录内存
freeContacts();
return 0;
}
```
阅读全文
相关推荐

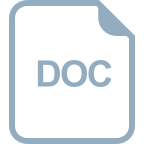
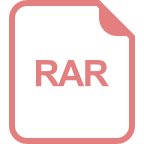
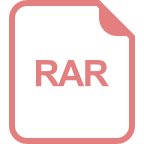
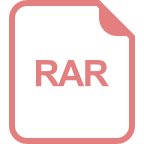
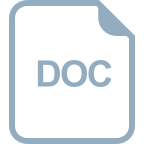
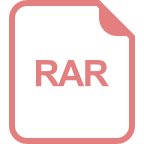
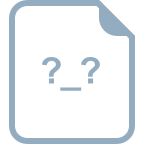
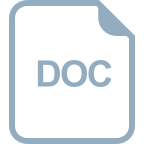
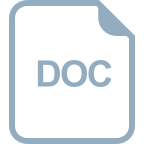
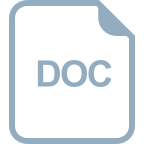
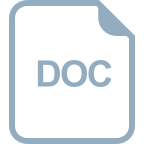
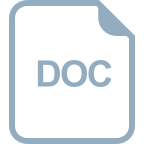
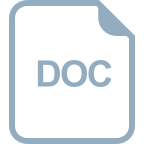

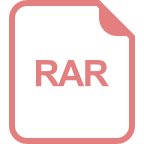