springboot基于API的redis缓存实现
时间: 2024-12-10 11:37:51 浏览: 15
Spring Boot 中基于 API 的 Redis 缓存实现通常通过 Spring Data Redis 和 Spring Cache 模块配合完成。以下是基本步骤:
1. **添加依赖**:
在 Maven 或 Gradle 项目的 `pom.xml` 或 `build.gradle` 文件中添加 Spring Data Redis 和 Spring Actuator (用于监控) 的依赖。
```xml
<!-- Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<!-- Gradle -->
implementation 'org.springframework.boot:spring-boot-starter-data-redis'
```
2. **配置Redis**:
在 `application.properties` 或 `application.yml` 配置文件中设置 Redis 连接信息,例如主机、端口、密码等。
```properties
spring.redis.host=your-redis-host
spring.redis.port=6379
spring.redis.password=your-password
```
3. **使用`@Cacheable`注解**:
使用 `@Cacheable` 注解标记需要从 Redis 缓存获取数据的方法。如果数据在缓存中,则直接返回,否则计算并存储到缓存中。
```java
@GetMapping("/cache-api")
@Cacheable(value = "myCache", key = "#id")
public Object getFromCacheById(@PathVariable Long id) {
// 实现你的业务逻辑,并假设它会查询数据库
return yourService.findById(id);
}
```
4. **启用Spring Cache管理器**:
如果你想自定义缓存策略或者清除缓存,可以在 `application.properties` 中启用 Spring Cache 管理器,然后创建一个实现 `CacheManager` 接口的 Bean。
5. **使用`@CacheEvict`和`@CachePut`注解**:
- `@CacheEvict`:用于从缓存中删除指定键值对。
- `@CachePut`:用于更新已存在的缓存项。
6. **监控缓存状态**:
通过启动 Spring Boot 自带的 Actuator 应用程序,可以访问 `/actuator/cache` URL 来查看 Redis 缓存的详细信息。
阅读全文
相关推荐
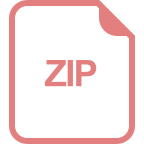
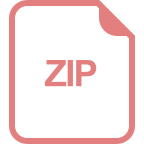
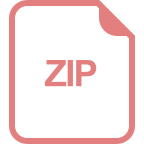
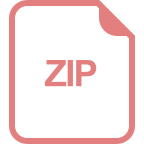




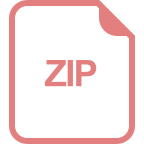
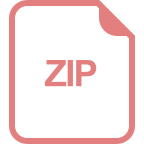
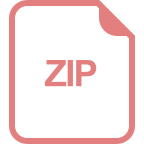
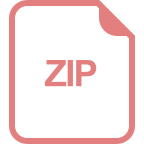
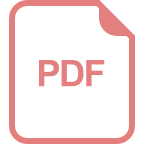
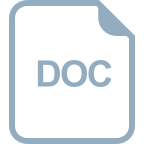
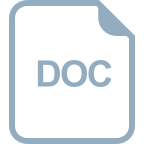
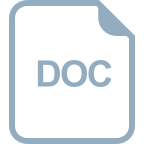


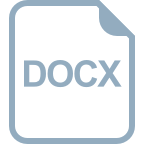