当前开发一个新语言、新中间件或程序框架时,基于充分利用多核CPU能力等方面的考虑,多采用事件循环处理模型+回调函数的设计方式,使用该模型的示例代码和预期输出详见下表。针对该模型,给出Trigger类及相关类的设计和实现,使得输出能够符合预期结果。c++
时间: 2024-11-12 14:42:12 浏览: 7
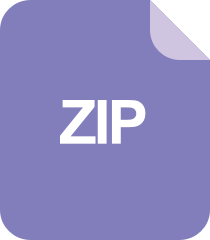
Node.js-Back2Front基于Express和XTemplate的模块化开发框架
在现代开发中,事件循环处理模型配合回调函数常用于构建高性能的并发系统,特别是当涉及到多线程或多进程处理大量异步任务时。例如,在C++中,我们可以使用`std::thread`, `std::async`, 或者像Boost.Asio这样的库来实现这种设计。
首先,让我们定义一个基础的`EventLoop`类,它会维护一个任务队列,并提供添加任务和执行任务的功能:
```cpp
#include <queue>
#include <functional>
class EventLoop {
public:
void post_task(std::function<void()> task) {
tasks_.push_back(task);
if (is_running_) {
process_tasks();
}
}
void start() {
is_running_ = true;
thread_ = std::thread(process_tasks);
}
void stop() {
is_running_ = false;
// Wait for the thread to finish processing current tasks
while (!tasks_.empty()) {
process_tasks();
}
thread_.join();
}
private:
bool is_running_ = false;
std::queue<std::function<void()>> tasks_;
std::thread thread_;
void process_tasks() {
while (is_running_ && !tasks_.empty()) {
tasks_.front()(); // Execute the next task
tasks_.pop(); // Remove completed task
}
}
};
```
然后我们定义`Trigger`类,它可以注册回调并触发它们:
```cpp
class Trigger {
public:
explicit Trigger(EventLoop& event_loop)
: event_loop_(event_loop) {}
void register_callback(const std::string& id, std::function<void()> callback) {
callbacks_[id] = callback;
}
void trigger_event(const std::string& id) {
auto it = callbacks_.find(id);
if (it != callbacks_.end()) {
event_loop_.post_task([=]() mutable { it->second(); });
} else {
std::cerr << "No such event ID: " << id << std::endl;
}
}
private:
EventLoop& event_loop_;
std::unordered_map<std::string, std::function<void()>> callbacks_;
};
```
在这个例子中,当你创建一个`Trigger`实例并将其绑定到`EventLoop`上,你可以通过`trigger_event`方法启动一个异步操作。每个注册的回调会在`EventLoop`的下一个空闲时间点被执行。
预期输出和示例:
- 用户创建`Trigger`对象并关联`EventLoop`
- 用户注册回调函数(如处理数据接收、文件读取等)
- 用户调用`trigger_event`发起一个异步操作(如网络请求)
- 回调函数在`EventLoop`空闲时执行,处理响应或更新UI
相关问题--
1. 为什么事件循环模型适合多核环境?
2. 如何保证在`EventLoop`停止时所有任务都已完成?
3. 使用`std::async`代替自定义事件循环有何优缺点?
阅读全文
相关推荐
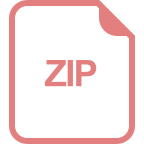
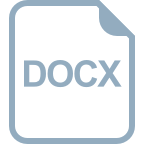
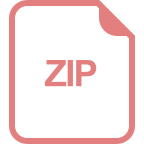
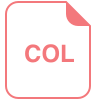
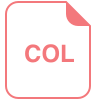
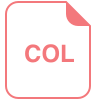
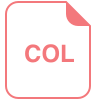
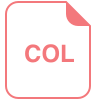
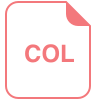
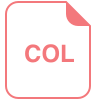
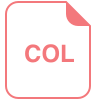
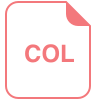
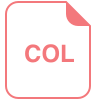
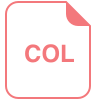
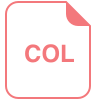
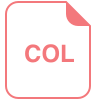