模拟退火的SAW板材开料优化C#代码
时间: 2023-10-19 19:22:39 浏览: 86
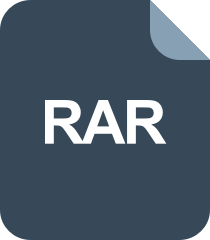
suanfa.rar_模拟退火算法 代码
以下是一个简单的C#代码示例,用于使用模拟退火算法进行SAW板材开料优化:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace SAWPlateOptimization
{
class Program
{
static void Main(string[] args)
{
// 初始化板材和零件列表
Plate plate = new Plate(200, 100); // 长200,宽100的板材
List<Part> parts = new List<Part>();
parts.Add(new Part(40, 20)); // 零件1,长40,宽20
parts.Add(new Part(30, 30)); // 零件2,长30,宽30
parts.Add(new Part(50, 10)); // 零件3,长50,宽10
// 初始化模拟退火参数
double temperature = 100;
double coolingRate = 0.03;
int numIterations = 1000;
// 使用模拟退火算法进行优化
PlateOptimizer optimizer = new PlateOptimizer(plate, parts);
Plate bestPlate = optimizer.Optimize(temperature, coolingRate, numIterations);
// 输出结果
Console.WriteLine("最优解:");
Console.WriteLine(bestPlate);
Console.ReadLine();
}
}
// 零件类
class Part
{
public double Length { get; set; }
public double Width { get; set; }
public Part(double length, double width)
{
this.Length = length;
this.Width = width;
}
public override string ToString()
{
return "零件(" + this.Length + " x " + this.Width + ")";
}
}
// 板材类
class Plate
{
public double Length { get; set; }
public double Width { get; set; }
public Plate(double length, double width)
{
this.Length = length;
this.Width = width;
}
public override string ToString()
{
return "板材(" + this.Length + " x " + this.Width + ")";
}
}
// 板材优化器类
class PlateOptimizer
{
private Plate plate;
private List<Part> parts;
public PlateOptimizer(Plate plate, List<Part> parts)
{
this.plate = plate;
this.parts = parts;
}
// 模拟退火算法进行优化
public Plate Optimize(double startingTemperature, double coolingRate, int numIterations)
{
Plate currentPlate = new Plate(plate.Length, plate.Width);
Plate bestPlate = new Plate(plate.Length, plate.Width);
// 随机生成初始解
foreach (Part part in parts)
{
currentPlate.PlacePartRandomly(part);
}
bestPlate.Copy(currentPlate);
double temperature = startingTemperature;
for (int i = 0; i < numIterations; i++)
{
// 生成新解
Plate newPlate = new Plate(plate.Length, plate.Width);
foreach (Part part in parts)
{
newPlate.PlacePartRandomly(part);
}
// 计算成本函数差异
double currentCost = currentPlate.GetCost();
double newCost = newPlate.GetCost();
double costDifference = newCost - currentCost;
// 判断是否接受新解
if (costDifference < 0 || Math.Exp(-costDifference / temperature) > new Random().NextDouble())
{
currentPlate.Copy(newPlate);
if (currentCost < bestPlate.GetCost())
{
bestPlate.Copy(currentPlate);
}
}
// 降温
temperature *= 1 - coolingRate;
}
return bestPlate;
}
}
// 扩展方法类
static class Extensions
{
// 在板材上随机放置零件
public static void PlacePartRandomly(this Plate plate, Part part)
{
bool placed = false;
while (!placed)
{
double x = new Random().NextDouble() * (plate.Length - part.Length);
double y = new Random().NextDouble() * (plate.Width - part.Width);
// 检查是否与已放置的零件重叠
bool overlap = false;
foreach (Part placedPart in plate.PlacedParts)
{
if (x + part.Length > placedPart.X && x < placedPart.X + placedPart.Length &&
y + part.Width > placedPart.Y && y < placedPart.Y + placedPart.Width)
{
overlap = true;
break;
}
}
if (!overlap)
{
part.X = x;
part.Y = y;
plate.PlacedParts.Add(part);
placed = true;
}
}
}
// 获取板材优化成本函数(即未被覆盖的面积)
public static double GetCost(this Plate plate)
{
double totalArea = plate.Length * plate.Width;
double coveredArea = 0;
foreach (Part part in plate.PlacedParts)
{
coveredArea += part.Length * part.Width;
}
return totalArea - coveredArea;
}
// 复制板材
public static void Copy(this Plate plate, Plate other)
{
plate.Length = other.Length;
plate.Width = other.Width;
plate.PlacedParts = new List<Part>(other.PlacedParts);
}
// 复制零件
public static void Copy(this Part part, Part other)
{
part.Length = other.Length;
part.Width = other.Width;
part.X = other.X;
part.Y = other.Y;
}
}
}
```
在这个示例中,我们定义了一个 `Plate` 类表示板材,一个 `Part` 类表示零件,以及一个 `PlateOptimizer` 类实现了模拟退火算法进行优化。我们还定义了一些扩展方法,用于计算成本函数、复制对象等。
在 `PlateOptimizer` 的 `Optimize` 方法中,我们使用模拟退火算法进行优化。我们首先随机生成一个初始解,并将其设置为当前最好的解。然后,我们在每次迭代中生成一个新解,并计算两个解的成本函数差异。如果新解更好,则直接接受它。否则,我们按照概率接受它,概率由当前温度和成本函数差异决定。最后,我们将温度降低一定比例,开始下一次迭代。
在 `Plate` 的扩展方法中,我们实现了在板材上随机放置零件、计算成本函数、复制对象等操作。
这只是一个简单的示例,实际应用中可能需要更复杂的算法和数据结构。但是,这个示例可以作为一个起点,帮助您开始使用模拟退火算法进行SAW板材开料优化。
阅读全文
相关推荐

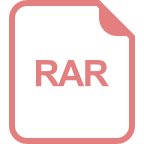
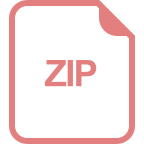
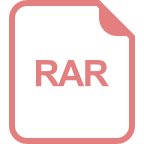
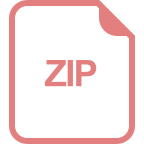
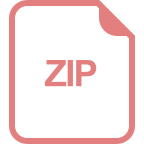
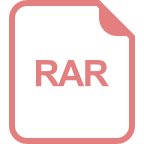
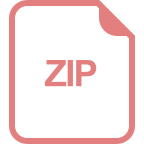
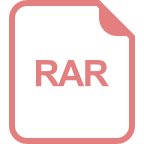
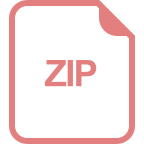