tcp聊天室Java代码
时间: 2023-07-03 21:28:08 浏览: 55
以下是一个简单的 TCP 聊天室 Java 代码示例:
Server.java:
```java
import java.net.*;
import java.io.*;
public class Server {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(12345);
System.out.println("Server started.");
while (true) {
Socket clientSocket = serverSocket.accept();
System.out.println("Client connected.");
Thread t = new Thread(new ClientHandler(clientSocket));
t.start();
}
}
private static class ClientHandler implements Runnable {
private Socket clientSocket;
private BufferedReader in;
private PrintWriter out;
public ClientHandler(Socket socket) {
this.clientSocket = socket;
}
public void run() {
try {
in = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
out = new PrintWriter(clientSocket.getOutputStream(), true);
out.println("Welcome to the server!");
String inputLine;
while ((inputLine = in.readLine()) != null) {
System.out.println("Client: " + inputLine);
out.println("Server: " + inputLine);
}
in.close();
out.close();
clientSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
Client.java:
```java
import java.net.*;
import java.io.*;
public class Client {
public static void main(String[] args) throws IOException {
Socket socket = new Socket("localhost", 12345);
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
String inputLine;
while ((inputLine = in.readLine()) != null) {
System.out.println(inputLine);
BufferedReader consoleIn = new BufferedReader(new InputStreamReader(System.in));
String consoleInput = consoleIn.readLine();
out.println(consoleInput);
}
in.close();
out.close();
socket.close();
}
}
```
该示例中的 Server 类创建一个服务器套接字,并在接受客户端连接后启动一个新的线程来处理该客户端的消息。ClientHandler 类实现了 Runnable 接口,用于读取客户端发送的消息并将其发送回客户端。
Client 类连接到服务器套接字并读取欢迎消息。它然后等待用户输入并将其发送到服务器。服务器将读取该消息并将其发送回客户端。该循环将继续直到客户端关闭连接。
请注意,此示例仅用于演示目的,并不是一个完整的聊天室应用程序。它需要进一步的开发和测试,以确保安全性和可靠性。
相关推荐
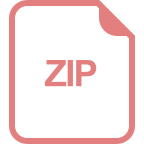














