前端数组转树的思路及代码解释
时间: 2023-08-27 11:03:59 浏览: 88
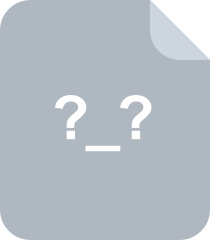
01-数组转树.md
前端数组转树的思路和后端类似,都是采用递归的方式将数组转换成树。具体思路如下:
1. 定义树节点的数据结构。
2. 定义递归函数,接收一个数组和一个起始索引作为参数。
3. 在递归函数内部,创建一个树节点,并将数组中的元素赋值给该节点的值。
4. 判断该节点是否有左子节点,如果有,递归调用函数,将左子树的数组和左子节点的索引作为参数传递给函数,并将返回值作为当前节点的左子树指针。
5. 判断该节点是否有右子节点,如果有,递归调用函数,将右子树的数组和右子节点的索引作为参数传递给函数,并将返回值作为当前节点的右子树指针。
6. 返回当前节点。
下面是一个示例代码:
```javascript
class TreeNode {
constructor(val) {
this.val = val;
this.left = null;
this.right = null;
}
}
function arrayToTree(nums, start) {
if (start >= nums.length || nums[start] === null) {
return null;
}
const root = new TreeNode(nums[start]);
root.left = arrayToTree(nums, 2 * start + 1);
root.right = arrayToTree(nums, 2 * start + 2);
return root;
}
// 示例用法
const nums = [1, 2, 3, null, 4, 5, 6];
const root = arrayToTree(nums, 0);
```
在示例代码中,我们将数组 `[1, 2, 3, null, 4, 5, 6]` 转换为如下的二叉树:
```
1
/ \
2 3
/ \
4 5
/
6
```
阅读全文
相关推荐
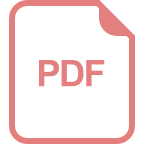
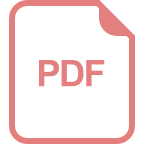















