unordered_set.find()
时间: 2023-12-10 11:31:30 浏览: 71
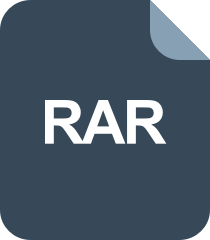
stl_code.rar_STL vector_hash_stl set code_vector_vector stl
unordered_set.find() 是C++中的一个函数,用于在无序集合(unordered_set)中查找指定元素的位置。它返回一个迭代器,指向包含该元素的位置,如果集合中不存在该元素,则返回一个指向集合末尾的迭代器。
例如,假设我们有一个无序集合 nums,我们可以使用 unordered_set.find() 函数来查找元素 5 是否存在:
```cpp
#include <iostream>
#include <unordered_set>
int main() {
std::unordered_set<int> nums = {1, 3, 5, 7, 9};
auto it = nums.find(5);
if (it != nums.end()) {
std::cout << "Element 5 found!" << std::endl;
} else {
std::cout << "Element 5 not found." << std::endl;
}
return 0;
}
```
输出结果为 "Element 5 found!",表示元素 5 存在于集合中。
阅读全文
相关推荐
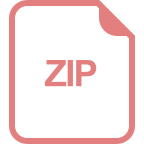
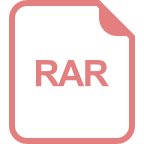















