如何使用python 将window 某个文件夹A以及其内部所有文件及文件夹 递归的 移动到 Centos7系统某文件夹B下,包括A文件夹
时间: 2023-06-18 14:05:47 浏览: 50
你可以使用Python的shutil模块来实现文件或文件夹的移动。
首先,需要在Centos7系统上设置好共享文件夹B,确保可以通过网络访问这个文件夹。
然后,在Python中,你可以使用以下代码将Windows系统的文件夹A递归地移动到Centos7系统的文件夹B下:
```python
import shutil
# Windows系统的文件夹A路径
windows_path = r'C:\path\to\folder\A'
# Centos7系统的文件夹B路径(需要先挂载共享文件夹)
centos_path = '/mnt/B'
# 使用shutil模块递归地移动文件夹A到文件夹B下
shutil.move(windows_path, centos_path)
```
这个代码会将Windows系统的文件夹A及其内部所有文件和文件夹递归地移动到Centos7系统的文件夹B下。需要注意的是,文件夹B需要先通过网络共享设置好,并且需要在Python中使用正确的路径访问。
相关问题
如何使用python 通过SSH 将window 某个文件夹A以及其内部所有文件及文件夹 递归的 移动到 Centos7系统某文件夹B下,包括A文件夹
你可以使用 Paramiko 模块在 Python 中执行 SSH 命令,并使用 SCP 将文件夹及其内容复制到 CentOS 7 中。以下是一个简单的示例:
```python
import paramiko
import os
# SSH 连接信息
host = "192.168.1.100"
port = 22
username = "user"
password = "password"
# 本地文件夹路径和远程文件夹路径
local_path = r"C:\Users\username\Documents\A"
remote_path = "/home/user/B"
# 创建 SSH 客户端
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(host, port, username, password)
# 创建 SCP 客户端
scp = ssh.open_sftp()
# 递归复制本地文件夹到远程服务器
for root, dirs, files in os.walk(local_path):
for file in files:
local_file = os.path.join(root, file)
remote_file = os.path.join(remote_path, os.path.relpath(local_file, local_path))
scp.put(local_file, remote_file)
# 关闭 SCP 客户端和 SSH 客户端
scp.close()
ssh.close()
```
在上面的代码中,我们使用 paramiko 模块创建了一个 SSH 连接,并设置了本地文件夹路径和远程文件夹路径。然后,我们使用 os.walk 函数遍历本地文件夹及其子文件夹和文件,并使用 SCP 将文件和文件夹复制到远程服务器上。最后,我们关闭了 SCP 客户端和 SSH 客户端。
window 下使用python paramiko ssh 将文件夹移动到Centos下
你可以使用 Paramiko 库来在 Python 中进行 SSH 操作,从而将文件夹从 Windows 移动到 CentOS。以下是一个基本的示例:
```python
import paramiko
import os
# 配置 SSH 连接参数
host = "your_host_ip"
port = 22
username = "your_username"
password = "your_password"
# 建立 SSH 连接
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(host, port, username, password)
# 在 CentOS 上创建目标文件夹
remote_dir = "/path/to/remote/directory"
stdin, stdout, stderr = ssh.exec_command(f"mkdir -p {remote_dir}")
# 获取本地文件夹的路径
local_dir = os.path.abspath("your/local/directory")
# 使用 SFTP 将本地文件夹上传到 CentOS
sftp = ssh.open_sftp()
sftp.put(local_dir, remote_dir, recursive=True)
# 关闭连接
sftp.close()
ssh.close()
```
这段代码完成了以下操作:
1. 建立了一个 SSH 连接,使用了你的主机 IP、SSH 端口、用户名和密码。
2. 在 CentOS 上创建了一个目标文件夹,如果已经存在则忽略。
3. 获取了本地文件夹的绝对路径。
4. 使用 SFTP 上传了本地文件夹到 CentOS,递归上传子文件夹和文件。
5. 关闭了 SSH 连接。
请注意替换示例代码中的 `your_host_ip`、`your_username`、`your_password`、`/path/to/remote/directory` 和 `your/local/directory` 为你自己的实际值。
相关推荐
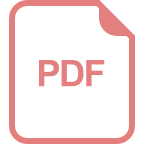












