java 用多线程写导出多个sheet页Excel功能 带有代码注释
时间: 2024-02-19 14:28:11 浏览: 30
下面是一个简单的 Java 多线程导出多个 Sheet 页 Excel 的示例代码,其中包含了注释说明:
```java
import java.io.FileOutputStream;
import java.util.ArrayList;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.CreationHelper;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExportExcelWithMultiThreads {
// 定义线程数
private static final int THREAD_COUNT = 3;
// 定义每个线程需要导出的行数
private static final int ROW_COUNT_PER_THREAD = 100000;
public static void main(String[] args) throws Exception {
// 创建工作簿
Workbook workbook = new XSSFWorkbook();
// 创建样式
CellStyle dateCellStyle = workbook.createCellStyle();
CreationHelper creationHelper = workbook.getCreationHelper();
dateCellStyle.setDataFormat(creationHelper.createDataFormat().getFormat("yyyy-MM-dd HH:mm:ss"));
// 创建多个 sheet 页
List<Sheet> sheets = new ArrayList<>();
for (int i = 0; i < THREAD_COUNT; i++) {
Sheet sheet = workbook.createSheet("Sheet" + (i + 1));
sheets.add(sheet);
}
// 创建多个线程并启动
List<Thread> threads = new ArrayList<>();
for (int i = 0; i < THREAD_COUNT; i++) {
final int threadNumber = i + 1;
Thread thread = new Thread(() -> {
// 计算本线程需要导出的起始行号和结束行号
int startRowNumber = (threadNumber - 1) * ROW_COUNT_PER_THREAD + 1;
int endRowNumber = threadNumber * ROW_COUNT_PER_THREAD;
// 在本线程中导出数据
for (int j = startRowNumber; j <= endRowNumber; j++) {
Sheet sheet = sheets.get(threadNumber - 1);
Row row = sheet.createRow(j - 1);
Cell cell1 = row.createCell(0);
cell1.setCellValue("Name " + j);
Cell cell2 = row.createCell(1);
cell2.setCellValue(j);
Cell cell3 = row.createCell(2);
cell3.setCellValue(System.currentTimeMillis());
cell3.setCellStyle(dateCellStyle);
}
});
threads.add(thread);
thread.start();
}
// 等待所有线程结束
for (Thread thread : threads) {
thread.join();
}
// 将工作簿保存到文件
try (FileOutputStream fileOutputStream = new FileOutputStream("test.xlsx")) {
workbook.write(fileOutputStream);
}
}
}
```
在上述示例代码中,我们创建了 3 个 Sheet 页,并启动了 3 个线程,每个线程需要导出 10 万行数据。我们在每个线程中计算出本线程需要导出的起始行号和结束行号,并在此范围内导出数据。最后,我们等待所有线程结束后,将工作簿保存到文件中。
相关推荐
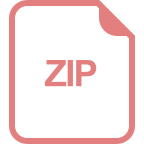
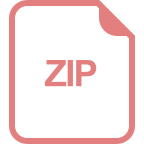
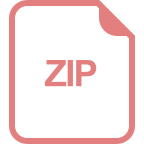














