用C语言写串口数据处理代码,RS422协议 波特率115200、数据位为8、停止位为1、无校验、帧格式:FF Len Type nByte CheckSum
时间: 2023-10-20 18:25:50 浏览: 96
下面是一个简单的C语言串口数据处理代码,可以用于处理RS422协议数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define FRAME_HEAD 0xFF // 帧头
#define FRAME_LEN_POS 1 // 帧长度位置
#define FRAME_TYPE_POS 2 // 帧类型位置
#define FRAME_DATA_POS 3 // 帧数据起始位置
#define BUF_SIZE 1024 // 缓冲区大小
int main(int argc, char *argv[]) {
int fd, n;
char buf[BUF_SIZE];
struct termios options;
// 打开串口设备文件
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("open");
exit(1);
}
// 设置串口参数
tcgetattr(fd, &options);
cfsetispeed(&options, B115200);
cfsetospeed(&options, B115200);
options.c_cflag |= CLOCAL | CREAD;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 1;
options.c_cc[VTIME] = 0;
tcsetattr(fd, TCSANOW, &options);
while (1) {
// 读取串口数据
n = read(fd, buf, BUF_SIZE);
if (n > 0) {
// 查找帧头
for (int i = 0; i < n; i++) {
if (buf[i] == FRAME_HEAD) {
// 判断帧长度是否正确
int len = buf[i + FRAME_LEN_POS];
if (len != n - i) {
break; // 帧长度不正确,跳过
}
// 计算校验和
char sum = 0;
for (int j = 0; j < len - 1; j++) {
sum += buf[i + j];
}
if (sum != buf[i + len - 1]) {
break; // 校验和错误,跳过
}
// 处理帧数据
char type = buf[i + FRAME_TYPE_POS];
char *data = &buf[i + FRAME_DATA_POS];
int nByte = len - FRAME_DATA_POS - 1;
// TODO: 处理接收到的数据
// 跳过已处理的数据
i += len - 1;
}
}
}
}
// 关闭串口设备文件
close(fd);
return 0;
}
```
上面的代码中,首先打开串口设备文件,然后设置串口参数,包括波特率、数据位、停止位、校验等。接着进入一个循环,不断读取串口数据,并在数据中查找帧头。如果找到帧头,就判断帧长度和校验和是否正确,并处理帧数据。最后跳过已处理的数据,继续处理下一帧数据。
阅读全文
相关推荐
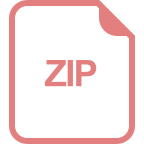
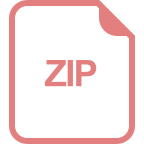
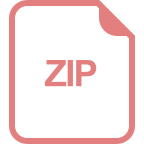
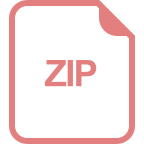
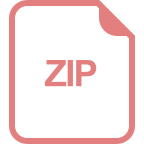
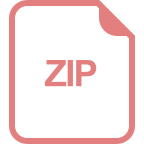
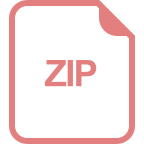