用C#写一段PS自动色调代码
时间: 2024-10-16 20:13:29 浏览: 7
在C#中,由于Photoshop是一款专业的图像处理软件,并非语言内置支持的库,所以直接编写PS自动化调整色调的代码通常是通过Adobe的Visual Studio SDK或者IronPython等工具配合使用。不过,如果你想要模拟类似操作,你可以使用.NET Framework中的System.Drawing命名空间来进行基本的颜色处理,如改变图片的亮度、对比度或色彩平衡。
下面是一个简单的示例,展示如何用C#创建一个方法来调整图片的整体色阶(亮度和对比度):
```csharp
using System.Drawing;
using System.Drawing.Imaging;
public static Bitmap AdjustTone(Bitmap originalImage, float brightness, float contrast)
{
// 创建一个新的Bitmap用于保存调整后的图像
Bitmap result = new Bitmap(originalImage.Width, originalImage.Height);
// 创建Graphics对象,准备绘制到新图像上
Graphics graphics = Graphics.FromImage(result);
// 设置颜色模式,以便进行像素级别的操作
ColorMatrix colorMatrix = new ColorMatrix(
new float[,]
{
{brightness, 0, 0, 0, 0},
{0, brightness, 0, 0, 0},
{0, 0, brightness, 0, 0},
{0, 0, 0, contrast, 0},
{0, 0, 0, 0, 1}
});
// 使用ColorMatrix类调整每个像素的颜色
ImageAttributes attributes = new ImageAttributes();
attributes.SetColorMatrix(colorMatrix);
graphics.DrawImage(originalImage, new Rectangle(0, 0, originalImage.Width, originalImage.Height), 0, 0, originalImage.Width, originalImage.Height, GraphicsUnit.Pixel, attributes);
return result;
}
// 调用示例
Bitmap inputImage = /* 从文件或流加载的原始图像 */;
Bitmap adjustedImage = AdjustTone(inputImage, /* 指定的亮度值 */, /* 指定的对比度值 */);
// 保存调整后的图像...
```
请注意,这只是一个非常基础的例子,实际的PS自动化需要对Photoshop对象模型有深入理解并可能涉及到COM Interop调用。此外,上述代码不会精确模拟出Photoshop的各种色调调整选项,如HSL、曲线或色彩校正等高级功能。
阅读全文
相关推荐
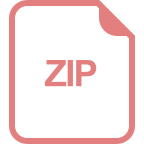
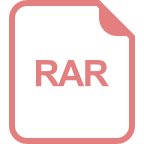
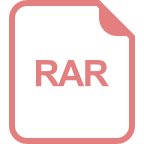
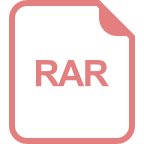
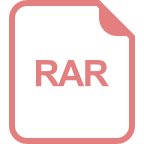
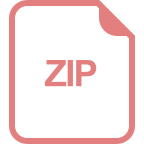
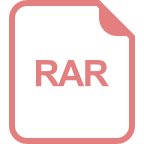
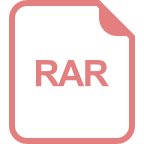
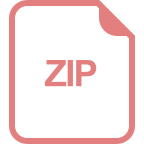
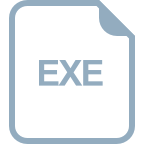
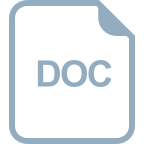
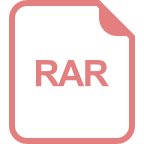
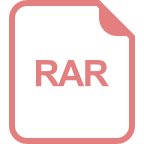
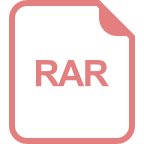
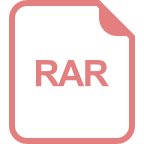