帮我写一段matlab用蚁群算法解决tsp问题的代码
时间: 2023-08-28 11:27:52 浏览: 156
好的,以下是一个简单的 Matlab 蚁群算法解决 TSP 问题的代码示例:
```matlab
% TSP 问题的数据
distances = [0 3 4 2 7;
3 0 4 6 3;
4 4 0 5 8;
2 6 5 0 6;
7 3 8 6 0];
num_cities = size(distances, 1);
% 蚁群算法参数设置
num_ants = 10; % 蚂蚁数量
alpha = 1; % 信息素重要程度因子
beta = 5; % 启发函数重要程度因子
evaporation_rate = 0.5; % 信息素蒸发率
pheromone_init = 1; % 初始信息素值
max_iterations = 100; % 最大迭代次数
% 初始化信息素矩阵
pheromone = pheromone_init * ones(num_cities, num_cities);
% 迭代求解 TSP
for iteration = 1:max_iterations
% 记录每个蚂蚁的路径和距离
paths = zeros(num_ants, num_cities);
distances_traveled = zeros(num_ants, 1);
% 让每只蚂蚁进行一次完整的 TSP 路径搜索
for ant = 1:num_ants
% 初始化当前蚂蚁的起始城市
current_city = randi(num_cities);
path = current_city;
% 进行剩余城市的遍历
for city_index = 2:num_cities
% 计算当前城市到各城市的信息素与启发函数值
pheromone_values = pheromone(current_city, :);
heuristic_values = 1 ./ distances(current_city, :);
probabilities = pheromone_values .^ alpha .* heuristic_values .^ beta;
probabilities(path) = 0; % 避免重复访问
% 根据概率选择下一个城市
probabilities = probabilities / sum(probabilities);
next_city = randsample(num_cities, 1, true, probabilities);
% 更新路径和距离
path = [path next_city];
distances_traveled(ant) = distances_traveled(ant) + distances(current_city, next_city);
% 更新当前城市
current_city = next_city;
end
% 回到起点城市并记录路径
path = [path path(1)];
paths(ant, :) = path;
distances_traveled(ant) = distances_traveled(ant) + distances(current_city, path(1));
end
% 计算每个城市的信息素更新量
delta_pheromone = zeros(num_cities, num_cities);
for ant = 1:num_ants
for city_index = 1:num_cities
from_city = paths(ant, city_index);
to_city = paths(ant, city_index + 1);
delta_pheromone(from_city, to_city) = delta_pheromone(from_city, to_city) + 1 / distances_traveled(ant);
end
end
% 更新信息素矩阵并进行信息素蒸发
pheromone = (1 - evaporation_rate) * pheromone + delta_pheromone;
end
% 输出最优路径及其距离
best_distance = Inf;
best_path = [];
for ant = 1:num_ants
if distances_traveled(ant) < best_distance
best_distance = distances_traveled(ant);
best_path = paths(ant, :);
end
end
fprintf('最优路径: %s\n', num2str(best_path));
fprintf('最优路径距离: %f\n', best_distance);
```
在代码中,我们使用了 `distances` 矩阵来存储 TSP 问题的距离数据,使用 `pheromone` 矩阵来存储城市间的信息素强度。在每次迭代中,我们让每只蚂蚁根据信息素强度和启发函数值选择下一个城市,并根据路径长度计算并更新信息素强度。最终输出最优路径及其距离。
阅读全文
相关推荐
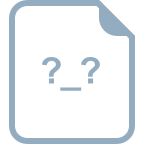

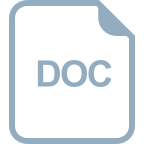
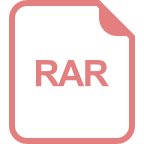
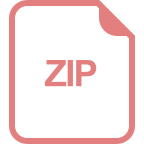
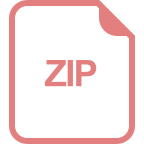
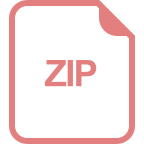
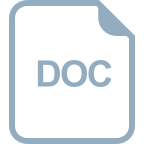
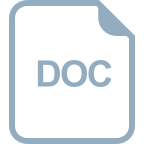
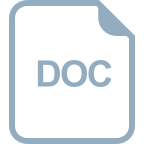
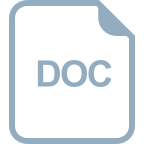
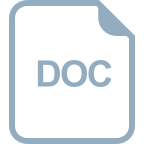
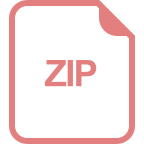
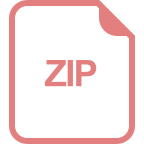
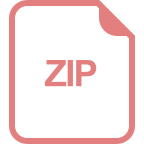
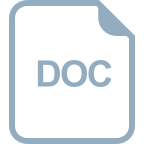