用python将电脑摄像机中的人脸截取出来,并保存在一个新的文件夹里
时间: 2024-05-08 10:17:21 浏览: 144
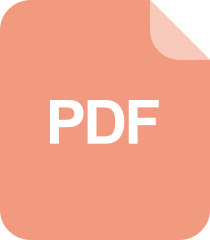
用Python批量把文件复制到另一个文件夹的实现方法

这个任务需要使用OpenCV和face_recognition库来实现。
首先,需要安装这两个库。可以使用以下命令:
```
pip install opencv-python
pip install face_recognition
```
然后,我们可以使用以下代码来实现将电脑摄像机中的人脸截取出来,并保存在一个新的文件夹里:
```python
import cv2
import face_recognition
import os
# 创建一个新的目录用于存储人脸图片
if not os.path.exists('faces'):
os.makedirs('faces')
# 打开电脑摄像机
video_capture = cv2.VideoCapture(0)
while True:
# 读取视频流中的帧
ret, frame = video_capture.read()
# 将帧转换为RGB颜色空间
rgb_frame = frame[:, :, ::-1]
# 使用face_recognition库识别帧中的所有人脸
face_locations = face_recognition.face_locations(rgb_frame)
# 遍历每个人脸位置,并将其截取出来存储到新的目录中
for face_location in face_locations:
top, right, bottom, left = face_location
face_image = frame[top:bottom, left:right]
cv2.imwrite('faces/face_{}.jpg'.format(len(os.listdir('faces'))), face_image)
# 显示帧
cv2.imshow('Video', frame)
# 按'q'键退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 关闭摄像机和窗口
video_capture.release()
cv2.destroyAllWindows()
```
运行代码后,程序会打开电脑摄像机,并开始识别视频流中的人脸。每当识别到一个人脸时,程序会将其截取出来并保存到新的目录中。按下'q'键后程序会退出循环,关闭摄像机和窗口。
注意,这个程序只会识别帧中的第一个人脸。如果需要识别多个人脸,需要对代码进行修改。
阅读全文
相关推荐
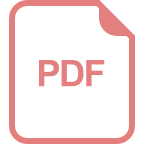
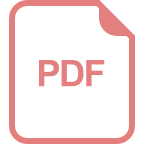














