c语言编程实现一元多项式加法运算,以(0,0)作为输入结束。
时间: 2023-06-01 11:02:16 浏览: 112
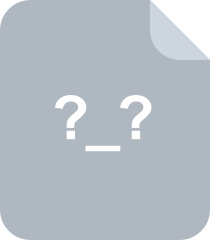
c语言实现的多项式加法

#include <stdio.h>
#define MAX_TERMS 100
typedef struct {
float coef; // 系数
int exp; // 指数
} Term;
void input_poly(Term poly[]);
void add_poly(const Term poly1[], const Term poly2[], Term result[]);
void print_poly(const Term poly[]);
int main() {
Term poly1[MAX_TERMS], poly2[MAX_TERMS], result[MAX_TERMS];
printf("请输入第一个多项式:\n");
input_poly(poly1);
printf("请输入第二个多项式:\n");
input_poly(poly2);
add_poly(poly1, poly2, result);
printf("两个多项式相加的结果为:\n");
print_poly(result);
return 0;
}
void input_poly(Term poly[]) {
int i = 0;
while (1) {
printf("请输入第%d项的系数和指数:", i + 1);
scanf("%f%d", &poly[i].coef, &poly[i].exp);
if (poly[i].coef == 0 && poly[i].exp == 0) {
break;
}
i++;
}
}
void add_poly(const Term poly1[], const Term poly2[], Term result[]) {
int i = 0, j = 0, k = 0;
while (poly1[i].coef != 0 || poly1[i].exp != 0 || poly2[j].coef != 0 || poly2[j].exp != 0) {
if (poly1[i].exp > poly2[j].exp) {
result[k] = poly1[i];
i++;
} else if (poly1[i].exp < poly2[j].exp) {
result[k] = poly2[j];
j++;
} else {
result[k].coef = poly1[i].coef + poly2[j].coef;
result[k].exp = poly1[i].exp;
i++;
j++;
}
k++;
}
result[k].coef = 0;
result[k].exp = 0;
}
void print_poly(const Term poly[]) {
int i = 0;
while (poly[i].coef != 0 || poly[i].exp != 0) {
if (i > 0 && poly[i].coef > 0) {
printf("+");
}
if (poly[i].coef != 1 && poly[i].coef != -1) {
printf("%.2f", poly[i].coef);
} else if (poly[i].coef == -1) {
printf("-");
}
if (poly[i].exp == 0) {
printf("%.2f", poly[i].coef);
} else if (poly[i].exp == 1) {
printf("x");
} else {
printf("x^%d", poly[i].exp);
}
i++;
}
printf("\n");
}
阅读全文
相关推荐
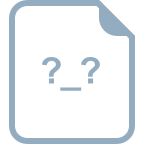
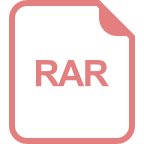
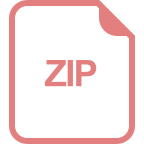
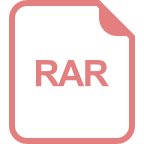
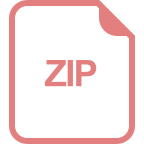