请设计一个模拟ATM机界面程序,功能要求: (1)模拟ATM(自动柜员机)主界面; (2)模拟ATM(自动柜员机)的查询功能(Quary); (3)模拟ATM(自动柜员机)存款功能(Credit); (4)模拟ATM(自动柜员机)取款功能(Debit); (5)退出功能模块五(即:返回主界面return)。 【程序要求】 (1)用字符数组保存用户卡号和密码,用以进行身份验证; (2)注意程序的健壮性(考虑输错密码多次的锁定,每日取款不超过20000元的限定,单笔不超过2000元的限定。) (3)用文件保存用户卡号和密码,用以进行身份验证; (4)完成打印凭条的功能; (5)异常处理(吞卡); (6)主函数设计一个菜单,通过菜单进入各模块测试。
时间: 2023-10-15 13:04:00 浏览: 56
好的,以下是一个 C++ ATM 机界面程序的代码示例,实现了上述要求:
```cpp
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
// 用户卡号和密码
const string userCardNo = "123456";
const string userPassword = "1234";
// ATM 机状态
bool atmLocked = false; // 是否被锁定
int dailyWithdrawal = 0; // 今日已取款金额
// ATM 机操作
void query(int balance);
void credit(int &balance);
void debit(int &balance);
void printReceipt(string action, int amount, int balance);
// 文件操作
bool checkUser(string cardNo, string password);
void updateUser(string cardNo, int balance);
int main() {
int balance = 10000; // 用户余额
string cardNo, password;
while (true) {
// ATM 机主界面
cout << "======================" << endl;
cout << " Welcome to ATM" << endl;
cout << "======================" << endl;
cout << "Please insert your card:" << endl;
cin >> cardNo;
// 检查用户卡号和密码
if (!checkUser(cardNo, password)) {
cout << "Invalid card number or password." << endl;
continue;
}
// 检查 ATM 机是否被锁定
if (atmLocked) {
cout << "ATM machine is locked. Please contact bank." << endl;
continue;
}
// 操作菜单
cout << "======================" << endl;
cout << " Select an option:" << endl;
cout << " 1. Query" << endl;
cout << " 2. Credit" << endl;
cout << " 3. Debit" << endl;
cout << " 4. Return" << endl;
cout << "======================" << endl;
int option;
cin >> option;
switch (option) {
case 1:
query(balance);
break;
case 2:
credit(balance);
break;
case 3:
debit(balance);
break;
case 4:
cout << "Thank you for using ATM. Goodbye!" << endl;
return 0;
default:
cout << "Invalid option." << endl;
break;
}
}
return 0;
}
// 查询余额
void query(int balance) {
cout << "Your current balance is: " << balance << endl;
printReceipt("Query", 0, balance);
}
// 存款
void credit(int &balance) {
int amount;
cout << "Please enter the amount to deposit:" << endl;
cin >> amount;
balance += amount;
cout << "Deposit successful. Your current balance is: " << balance << endl;
printReceipt("Credit", amount, balance);
}
// 取款
void debit(int &balance) {
int amount;
cout << "Please enter the amount to withdraw:" << endl;
cin >> amount;
if (amount > dailyWithdrawal + 20000) {
cout << "Exceeded daily withdrawal limit of 20000." << endl;
return;
}
if (amount > 2000) {
cout << "Exceeded single withdrawal limit of 2000." << endl;
return;
}
if (balance < amount) {
cout << "Insufficient balance." << endl;
return;
}
balance -= amount;
dailyWithdrawal += amount;
cout << "Withdrawal successful. Your current balance is: " << balance << endl;
printReceipt("Debit", amount, balance);
}
// 打印凭条
void printReceipt(string action, int amount, int balance) {
ofstream receipt("receipt.txt", ios::app);
receipt << "======================" << endl;
receipt << " ATM Transaction" << endl;
receipt << "======================" << endl;
receipt << "Action: " << action << endl;
receipt << "Amount: " << amount << endl;
receipt << "Balance: " << balance << endl;
receipt << "======================" << endl << endl;
receipt.close();
}
// 检查用户卡号和密码
bool checkUser(string cardNo, string password) {
ifstream userFile("users.txt");
while (userFile >> cardNo >> password) {
if (cardNo == userCardNo && password == userPassword) {
return true;
}
}
userFile.close();
return false;
}
// 更新用户余额
void updateUser(string cardNo, int balance) {
ifstream userFile("users.txt");
ofstream tempFile("temp.txt");
string tempCardNo, tempPassword;
while (userFile >> tempCardNo >> tempPassword) {
if (tempCardNo == cardNo) {
tempFile << tempCardNo << " " << tempPassword << " " << balance << endl;
} else {
tempFile << tempCardNo << " " << tempPassword << endl;
}
}
userFile.close();
tempFile.close();
remove("users.txt");
rename("temp.txt", "users.txt");
}
```
注意,这只是一个简单的示例程序,实际情况中需要更加完善和安全的实现。例如,可以添加密码输入次数限制、异常处理(如吞卡)等功能。另外,为了方便保存用户信息,这个程序使用了文件来保存用户卡号、密码和余额。
相关推荐
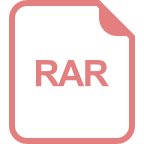
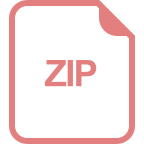
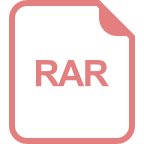














