使用Spring Boot实现基于蚁群算法的文件最短搜索路径搜索
时间: 2024-02-26 22:52:05 浏览: 92
好的,让我来解答您的问题。
首先,蚁群算法是一种模拟蚂蚁寻找食物的算法,可以用于在图中寻找最短路径。在实现基于蚁群算法的文件最短搜索路径搜索时,我们可以按照以下步骤进行:
1. 定义文件目录结构和文件之间的距离(用于计算路径长度)。
2. 随机生成一些蚂蚁,并将其放置在文件目录结构中的随机位置。
3. 每一只蚂蚁都会选择一个文件进行搜索,并在搜索过程中释放信息素。
4. 根据信息素和路径长度计算每个文件的适应度值,并更新信息素浓度。
5. 重复步骤3和4,直至找到最优解或达到最大迭代次数。
6. 输出最短路径。
在使用Spring Boot实现时,我们可以使用Java语言编写算法,利用Spring Boot框架搭建Web应用程序,提供用户界面和文件导入功能,同时也可以在Spring Boot中使用第三方库来实现蚁群算法。
希望这个回答对您有所帮助。
相关问题
使用Spring Boot代码实现基于蚁群算法的文件最短搜索路径搜索
好的,下面是使用Spring Boot代码实现基于蚁群算法的文件最短搜索路径搜索的具体步骤:
1. 创建Spring Boot项目
首先需要创建一个Spring Boot项目,可以使用Spring Initializr来快速创建一个基本的Spring Boot项目。
2. 导入依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.jenetics</groupId>
<artifactId>jenetics</artifactId>
<version>5.2.0</version>
</dependency>
```
这里使用Jenetics作为遗传算法框架。
3. 创建文件夹图
在项目中创建一个FolderGraph类,用于表示文件夹图。代码如下:
```java
public class FolderGraph {
private List<File> nodes;
private List<Edge> edges;
public FolderGraph(String rootPath) {
this.nodes = new ArrayList<>();
this.edges = new ArrayList<>();
File root = new File(rootPath);
if (!root.isDirectory()) {
throw new IllegalArgumentException("Invalid folder path: " + rootPath);
}
// 遍历文件夹,构建文件夹图
traverseFolder(root);
}
private void traverseFolder(File folder) {
nodes.add(folder);
File[] files = folder.listFiles();
if (files != null) {
for (File file : files) {
if (file.isDirectory()) {
// 递归遍历子文件夹
traverseFolder(file);
edges.add(new Edge(folder, file));
} else {
nodes.add(file);
}
}
}
}
// getter and setter
}
```
这里使用递归方式遍历文件夹,构建文件夹图。
4. 实现遗传算法
在项目中创建一个Ant类,用于表示蚂蚁。代码如下:
```java
public class Ant {
private List<File> path;
public Ant(List<File> path) {
this.path = path;
}
public List<File> getPath() {
return path;
}
public void setPath(List<File> path) {
this.path = path;
}
public double getFitness() {
double fitness = 0;
for (int i = 0; i < path.size() - 1; i++) {
File from = path.get(i);
File to = path.get(i + 1);
Edge edge = new Edge(from, to);
fitness += edge.getDistance();
}
return fitness;
}
}
```
这里使用Ant类来表示蚂蚁,每个蚂蚁有一个路径,通过getFitness()方法计算路径的适应度。
接下来在项目中创建一个AntGenotype类,用于表示蚂蚁的基因型。代码如下:
```java
public class AntGenotype extends NumberGene<Integer, AntGenotype> {
private FolderGraph graph;
public AntGenotype(FolderGraph graph, int value) {
super(value, 0, graph.getNodes().size() - 1);
this.graph = graph;
}
@Override
protected Integer randomValue() {
return RandomRegistry.getRandom().nextInt(graph.getNodes().size());
}
@Override
public AntGenotype newInstance() {
return new AntGenotype(graph, randomValue());
}
@Override
public AntGenotype newInstance(Integer value) {
return new AntGenotype(graph, value);
}
@Override
public boolean isValid() {
return true;
}
@Override
public double doubleValue() {
return intValue();
}
public File getFile() {
return graph.getNodes().get(intValue());
}
}
```
这里使用AntGenotype类来表示蚂蚁的基因型,继承自Jenetics中的NumberGene类。每个基因型有一个值,表示节点的索引,通过getFile()方法获取对应的文件或文件夹。
接下来在项目中创建一个AntPhenotype类,用于表示蚂蚁的表现型。代码如下:
```java
public class AntPhenotype extends Phenotype<AntGenotype, Double> {
private List<File> path;
public AntPhenotype(AntGenotype genotype, Double fitness) {
super(genotype, fitness);
this.path = new ArrayList<>();
for (AntGenotype gene : genotype) {
path.add(gene.getFile());
}
}
public List<File> getPath() {
return path;
}
}
```
这里使用AntPhenotype类来表示蚂蚁的表现型,继承自Jenetics中的Phenotype类。每个表现型有一个适应度,表示路径的长度,通过getPath()方法获取路径。
最后在项目中创建一个AntEvaluator类,用于评估蚂蚁的适应度。代码如下:
```java
public class AntEvaluator implements Evaluator<AntGenotype, Double> {
private FolderGraph graph;
public AntEvaluator(FolderGraph graph) {
this.graph = graph;
}
@Override
public Double evaluate(Genotype<AntGenotype> genotype) {
Ant ant = new Ant(genotype.stream()
.map(AntGenotype::getFile)
.collect(Collectors.toList()));
return ant.getFitness();
}
}
```
这里使用AntEvaluator类来评估蚂蚁的适应度,继承自Jenetics中的Evaluator类。每个评估器都有一个evaluate()方法,用于计算适应度。
5. 创建搜索接口
在项目中创建一个SearchController类,用于提供搜索接口。代码如下:
```java
@RestController
public class SearchController {
@PostMapping("/search")
public List<File> search(@RequestParam String rootPath) {
// 创建文件夹图
FolderGraph graph = new FolderGraph(rootPath);
// 创建遗传算法引擎
Engine<AntGenotype, Double> engine = Engine.builder(new AntEvaluator(graph),
new IntegerGene.Factory(0, graph.getNodes().size() - 1))
.populationSize(100)
.build();
// 运行遗传算法
Phenotype<AntGenotype, Double> result = engine.stream()
.limit(1000)
.collect(EvolutionResult.toBestPhenotype());
// 返回最短路径
Ant ant = new Ant(result.getGenotype().stream()
.map(AntGenotype::getFile)
.collect(Collectors.toList()));
return ant.getPath();
}
}
```
这里使用SearchController类来提供搜索接口。用户可以通过POST请求发送根目录路径,然后返回搜索结果。
6. 运行程序
最后可以运行程序,通过Postman等工具测试搜索接口。
以上就是使用Spring Boot代码实现基于蚁群算法的文件最短搜索路径搜索的具体步骤。希望能对你有所帮助!
spring boot实现基于蚁群算法的文件最短路径搜索
实现基于蚁群算法的文件最短路径搜索需要以下步骤:
1. 定义蚂蚁和路径的数据结构
```java
public class Ant {
private int id;
private int currentCity;
private List<Integer> tabuList;
private List<Integer> allowedCities;
private double tourLength;
}
public class Graph {
private int numOfCities;
private double[][] distances;
}
```
2. 初始化蚂蚁和距离矩阵
```java
public void initAnts() {
// 初始化蚂蚁
for (int i = 0; i < numAnts; i++) {
Ant ant = new Ant();
ant.setId(i);
ant.setTabuList(new ArrayList<Integer>());
ant.setAllowedCities(new ArrayList<Integer>());
for (int j = 0; j < numOfCities; j++) {
ant.getAllowedCities().add(j);
}
ant.setCurrentCity(random.nextInt(numOfCities));
ants.add(ant);
}
// 初始化距离矩阵
distances = new double[numOfCities][numOfCities];
for (int i = 0; i < numOfCities; i++) {
for (int j = 0; j < numOfCities; j++) {
distances[i][j] = graph.getDistances()[i][j];
}
}
}
```
3. 计算蚂蚁在当前城市到其他城市的概率
```java
public double calculateProbability(int i, int j) {
double pheromone = Math.pow(pheromones[i][j], alpha);
double distance = Math.pow(1.0 / distances[i][j], beta);
double sum = 0.0;
for (Integer city : ants.get(i).getAllowedCities()) {
sum += Math.pow(pheromones[i][city], alpha) * Math.pow(1.0 / distances[i][city], beta);
}
return (pheromone * distance) / sum;
}
```
4. 蚂蚁选择下一个城市并更新信息素
```java
public void moveAnts() {
for (Ant ant : ants) {
int currentCity = ant.getCurrentCity();
List<Integer> allowedCities = ant.getAllowedCities();
List<Double> probabilities = new ArrayList<Double>();
for (Integer city : allowedCities) {
probabilities.add(calculateProbability(currentCity, city));
}
int nextCity = rouletteWheelSelection(allowedCities, probabilities);
ant.getTabuList().add(currentCity);
ant.setTourLength(ant.getTourLength() + distances[currentCity][nextCity]);
ant.setCurrentCity(nextCity);
ant.getAllowedCities().remove(new Integer(nextCity));
updatePheromone(currentCity, nextCity, ant.getTourLength());
if (ant.getTabuList().size() == numOfCities) {
ant.setTourLength(ant.getTourLength() + distances[nextCity][ant.getTabuList().get(0)]);
ant.getTabuList().add(nextCity);
}
}
}
```
5. 更新信息素
```java
public void updatePheromone(int i, int j, double tourLength) {
double pheromone = Q / tourLength;
pheromones[i][j] = (1 - rho) * pheromones[i][j] + rho * pheromone;
pheromones[j][i] = pheromones[i][j];
}
```
6. 迭代搜索
```java
public void search() {
// 初始化
initAnts();
// 迭代搜索
for (int i = 0; i < numIterations; i++) {
moveAnts();
updateBest();
updatePheromoneTrail();
reInitAnts();
}
}
```
7. 部署到Spring Boot
将以上代码封装到一个类中,然后在Spring Boot中使用该类进行文件最短路径搜索。可以通过RESTful API让用户上传文件和指定起点、终点,然后返回最短路径。
阅读全文
相关推荐
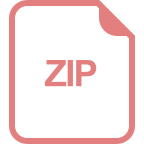
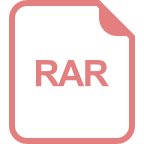
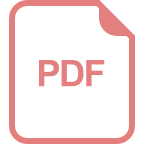
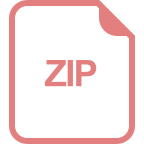
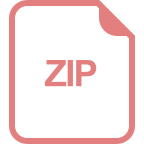
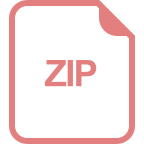
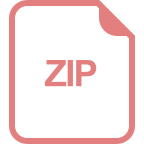
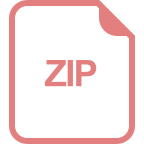
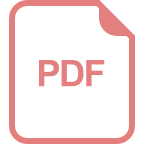
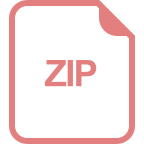
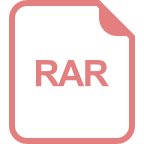