用代码实现一个demo,前端用ant-design-vue实现语音搜索框,后端用java调用百度语音识别实现语音转文字
时间: 2024-04-06 20:34:19 浏览: 59
好的,我可以提供一个简单的demo示例,来演示如何使用ant-design-vue实现语音搜索框,并结合Java后端调用百度语音识别API实现语音转文字的功能。
前端代码示例:
```vue
<template>
<div>
<a-input-search v-model="searchValue" :enter-button="true" @search="handleSearch">
<a-icon slot="suffix" v-if="!listening" type="search" @click="startListening" />
<a-icon slot="suffix" v-else type="loading" />
</a-input-search>
</div>
</template>
<script>
import { debounce } from 'lodash'
import { message } from 'ant-design-vue'
export default {
data() {
return {
searchValue: '',
listening: false
}
},
methods: {
handleSearch() {
// 处理搜索请求
console.log('搜索关键字:', this.searchValue)
},
startListening() {
// 开始语音识别
message.info('请开始说话')
this.listening = true
this.recognizeSpeech()
},
stopListening() {
// 结束语音识别
message.info('识别结束')
this.listening = false
},
recognizeSpeech: debounce(function() {
// 调用百度语音识别API
const token = '百度语音识别API的Token'
const url = `https://vop.baidu.com/pro_api?dev_pid=1536&cuid=xxxx&token=${token}`
const blob = new Blob([], { type: 'audio/wav' })
const formData = new FormData()
formData.append('audio', blob)
formData.append('len', '0')
formData.append('speech', '')
const headers = {
'Content-Type': 'application/json; charset=UTF-8'
}
this.$http.post(url, formData, { headers }).then(res => {
// 获取语音识别结果
console.log('语音识别结果:', res.data)
this.stopListening()
this.searchValue = res.data.result[0]
this.handleSearch()
}).catch(error => {
console.error(error)
message.error('语音识别失败,请重试')
this.stopListening()
})
}, 1000)
}
}
</script>
```
后端代码示例:
```java
public class BaiduSpeechRecognition {
private static final String URL = "https://vop.baidu.com/pro_api";
private static final String TOKEN = "百度语音识别API的Token";
private static final String CUID = "xxxx"; // 用户自定义ID
public static String recognizeSpeech(InputStream inputStream) throws IOException, URISyntaxException {
byte[] bytes = IOUtils.toByteArray(inputStream);
String base64Data = Base64.getEncoder().encodeToString(bytes);
HttpClient httpClient = HttpClientBuilder.create().build();
URIBuilder uriBuilder = new URIBuilder(URL);
uriBuilder.addParameter("dev_pid", "1536");
uriBuilder.addParameter("cuid", CUID);
uriBuilder.addParameter("token", TOKEN);
HttpPost httpPost = new HttpPost(uriBuilder.build());
httpPost.setHeader("Content-Type", "application/json; charset=UTF-8");
JSONObject json = new JSONObject();
json.put("speech", base64Data);
json.put("len", bytes.length);
httpPost.setEntity(new StringEntity(json.toJSONString(), Charset.forName("UTF-8")));
HttpResponse response = httpClient.execute(httpPost);
String result = EntityUtils.toString(response.getEntity());
JSONObject jsonObject = JSONObject.parseObject(result);
JSONArray jsonArray = jsonObject.getJSONArray("result");
return jsonArray.getString(0);
}
}
```
需要注意的是,以上示例代码仅供参考,并不完整,实际开发中还需要考虑异常处理、接口调用频率限制等问题。同时,还需要在百度语音识别API官网上申请API Key和Secret Key,并使用正确的Token进行接口调用。
阅读全文
相关推荐
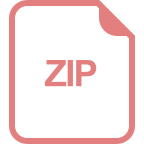
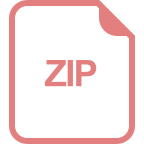
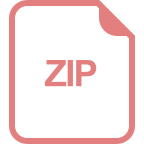
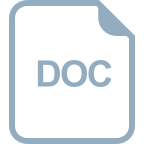
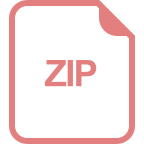
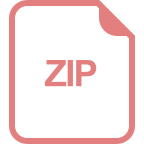
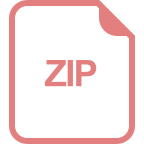
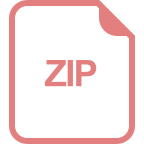
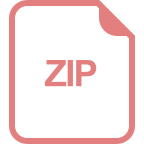


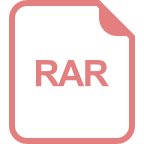
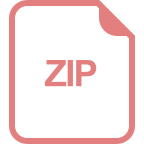
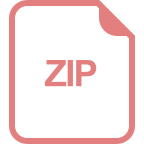