python求闰年
时间: 2023-07-01 08:26:54 浏览: 81
判断闰年的规则是:
1. 能被4整除但不能被100整除的年份是闰年(如2004年就是闰年,1900年不是闰年);
2. 能被400整除的是闰年(如2000年是闰年)
用Python编写判断闰年的代码如下:
```
def is_leap_year(year):
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
return True
else:
return False
```
其中,year为待判断的年份,函数返回True表示该年为闰年,返回False表示该年不是闰年。
相关问题
python求闰年个数
可以使用以下代码来计算某个年份范围内的闰年个数:
```python
def count_leap_years(start_year, end_year):
count = 0
for year in range(start_year, end_year+1):
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
count += 1
return count
```
其中,start_year 和 end_year 分别是需要计算的年份范围的起始年份和结束年份。该函数会遍历从 start_year 到 end_year 的所有年份,判断该年份是否为闰年,如果是,则计数器 count 加一。最后返回计数器的值即可。
Python中求闰年
Python中求闰年的方法有多种,以下是其中两种常用的方法:
1. 使用条件语句判断:根据闰年的定义,世纪年能被400整除的是闰年,非世纪年能被4整除的是闰年,否则是平年。可以使用if-else语句进行判断。
2. 使用datetime模块:Python中的datetime模块提供了date类,可以方便地获取年份信息。通过判断年份是否能被4整除,是否能被100整除但不能被400整除,来判断是否为闰年。
以下是两种方法的代码实现:
方法一:
def is_leap_year(year):
if year % 100 == 0:
if year % 400 == 0:
return True
else:
return False
else:
if year % 4 == 0:
return True
else:
return False
year = 2022
if is_leap_year(year):
print(year, "是闰年")
else:
print(year, "不是闰年")
方法二:
import datetime
def is_leap_year(year):
d = datetime.date(year, 1, 1)
if d.year % 4 == 0:
if d.year % 100 == 0:
if d.year % 400 == 0:
return True
else:
return False
else:
return True
else:
return False
year = 2022
if is_leap_year(year):
print(year, "是闰年")
else:
print(year, "不是闰年")
阅读全文
相关推荐
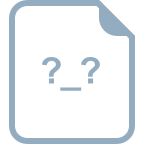
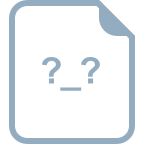











