实现以下内容,解释每一行的作用并写出去具体实现步骤: 1、stm32f103cbtx单片机串口发送时间到计算机,格式hh:mm:ss,每秒钟发一次; 2、计算发送SET_TIME hh:mm:ss到单片机,修改单片机时间。
时间: 2024-02-08 16:03:11 浏览: 130
以下是实现上述内容的具体步骤和代码解释:
1. 设置串口通信:
```c
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
// 使能串口和GPIO时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
// 配置USART1 Tx引脚为推挽输出
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1 Rx引脚为浮空输入
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1参数
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 使能USART1
USART_Cmd(USART1, ENABLE);
```
这段代码实现了对USART1串口的初始化和使能。其中,Tx引脚被配置为推挽输出,Rx引脚被配置为浮空输入。USART1的通信速率被设置为9600bps。
2. 发送时间到计算机:
```c
while (1) {
// 获取当前时间
RTC_GetTime(RTC_Format_BIN, &RTC_TimeStructure);
// 将时间格式化为hh:mm:ss
sprintf(buffer, "%02d:%02d:%02d", RTC_TimeStructure.RTC_Hours,
RTC_TimeStructure.RTC_Minutes, RTC_TimeStructure.RTC_Seconds);
// 发送时间到计算机
for (int i = 0; i < strlen(buffer); i++) {
USART_SendData(USART1, buffer[i]);
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET)
;
}
// 每秒钟发送一次
delay_ms(1000);
}
```
这段代码在一个while循环中不断获取当前时间,并将其格式化为hh:mm:ss的字符串格式。然后,使用USART1串口将该字符串发送到计算机。其中,USART_SendData()函数用于发送一个字节的数据,USART_GetFlagStatus()函数用于等待串口发送完毕,delay_ms()函数用于延时1秒。
3. 接收并修改时间:
```c
uint8_t time_buf[8];
while (1) {
// 等待接收到SET_TIME指令
while (USART_ReceiveData(USART1) != 'S')
;
if (USART_ReceiveData(USART1) == 'E' &&
USART_ReceiveData(USART1) == 'T' &&
USART_ReceiveData(USART1) == '_') {
// 读取时间
for (int i = 0; i < 8; i++) {
time_buf[i] = USART_ReceiveData(USART1);
}
// 将时间解析为RTC时间结构体
RTC_TimeStructure.RTC_Hours =
((time_buf[0] - '0') * 10) + (time_buf[1] - '0');
RTC_TimeStructure.RTC_Minutes =
((time_buf[3] - '0') * 10) + (time_buf[4] - '0');
RTC_TimeStructure.RTC_Seconds =
((time_buf[6] - '0') * 10) + (time_buf[7] - '0');
// 设置RTC时间
RTC_SetTime(RTC_Format_BIN, &RTC_TimeStructure);
}
}
```
这段代码在一个while循环中等待接收到SET_TIME指令,然后读取指令中的时间数据并解析为RTC时间结构体。最后,使用RTC_SetTime()函数将单片机的时间设置为接收到的时间。其中,USART_ReceiveData()函数用于接收一个字节的数据。
阅读全文
相关推荐














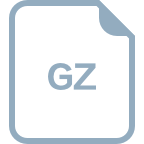

