Collections.sort()自定义排序用法举例
时间: 2023-06-02 21:04:03 浏览: 123
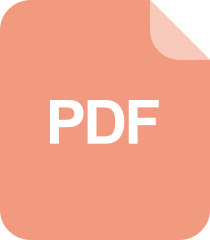
list泛型自定义排序示例
当使用Collections.sort()方法进行自定义排序时,需要实现接口Comparator并重写compare()方法。以下是一个例子:
假设我们有一个类Person,其中包含姓名和年龄两个属性:
```
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
现在我们想要按照年龄从小到大排序,可以这样实现:
```
List<Person> personList = new ArrayList<>();
personList.add(new Person("Jack", 25));
personList.add(new Person("Lucy", 18));
personList.add(new Person("Tom", 30));
// 自定义排序
Collections.sort(personList, new Comparator<Person>() {
@Override
public int compare(Person o1, Person o2) {
return o1.getAge() - o2.getAge();
}
});
// 输出排序结果
for (Person person : personList) {
System.out.println(person.getName() + ":" + person.getAge());
}
```
输出结果:
```
Lucy:18
Jack:25
Tom:30
```
在这个例子中,我们重写了compare()方法,实现了按照年龄从小到大排序。然后使用Collections.sort()方法对personList进行排序并输出结果。
阅读全文
相关推荐
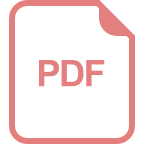
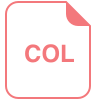
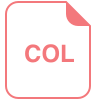
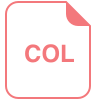
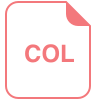
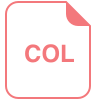
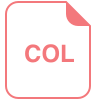


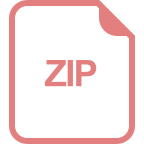
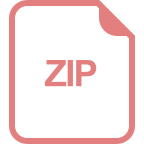
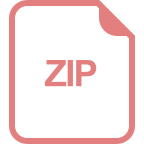
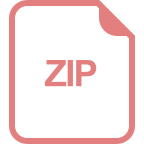
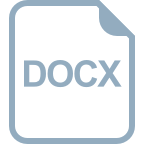
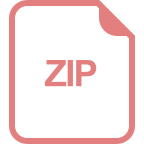
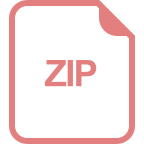
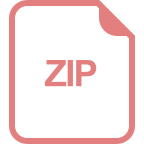