结合链表的基本运算,完成两个及以上多项式合并同类项实验 。 P1 2x+3x7+5x” P2 20x+4x+5元+90 S:20x+6x=+58+4x7×5+0 用c语言写写一个整的代码
时间: 2024-11-13 22:26:31 浏览: 8
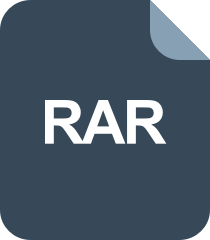
基于C++与链表的两个一元多项式的基本运算(加法、减法、 乘法和求导运算)

在C语言中,我们可以创建一个结构体来表示多项式的每个项,并利用链表的数据结构来存储多项式。这里是一个简单的示例,演示如何合并两个多项式并去除重复项:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Term {
int coefficient;
int exponent;
struct Term* next;
} Term;
// 初始化链表头结点
Term* createTerm(int coef, int exp) {
Term* new_term = (Term*)malloc(sizeof(Term));
new_term->coefficient = coef;
new_term->exponent = exp;
new_term->next = NULL;
return new_term;
}
// 合并两个多项式并去重
Term* mergePolynomials(Term* poly1, Term* poly2) {
// 遍历poly2
while (poly2 != NULL) {
int merged = 0; // 标记是否已添加到结果中
Term* current = poly1;
// 检查poly1中的每一项是否需要更新
while (current != NULL && !merged) {
if (current->exponent == poly2->exponent &&
current->coefficient + poly2->coefficient > 0) { // 如果系数相加大于0说明可以合并
current->coefficient += poly2->coefficient;
merged = 1;
} else {
current = current->next;
}
}
// 如果poly1中未找到合适的位置,将poly2的项插入
if (!merged) {
current = createTerm(poly2->coefficient, poly2->exponent);
if (poly1 == NULL) {
poly1 = current;
} else {
Term* last = poly1;
while (last->next != NULL) {
last = last->next;
}
last->next = current;
}
}
poly2 = poly2->next;
}
return poly1;
}
// 打印多项式
void printPolynomial(Term* poly) {
if (poly == NULL) {
printf("0\n");
return;
}
Term* temp = poly;
while (temp != NULL) {
printf("%dx^%d", temp->coefficient, temp->exponent);
if (temp->next != NULL) {
printf("+");
}
temp = temp->next;
}
printf("\n");
}
int main() {
// 定义两个多项式
Term* poly1 = createTerm(2, 1); // 2x + 3x^7 + 5x
poly1->next = createTerm(3, 7);
poly1->next->next = createTerm(5, 1);
Term* poly2 = createTerm(20, 1); // 20x + 4x + 5x^4 + 90
poly2->next = createTerm(4, 1);
poly2->next->next = createTerm(5, 4);
poly2->next->next->next = createTerm(90, 0); // 90表示常数项
// 合并多项式
Term* result = mergePolynomials(poly1, poly2);
// 打印结果
printf("合并后的多项式:\n");
printPolynomial(result);
return 0;
}
```
这个程序首先初始化了两个多项式,然后通过`mergePolynomials`函数合并它们并去除重复项。最后,`printPolynomial`函数用于打印合并后的多项式。
阅读全文
相关推荐
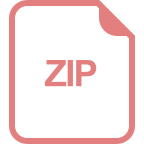
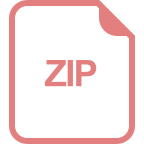
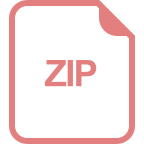
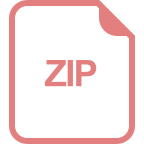
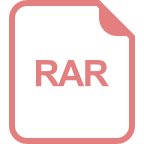
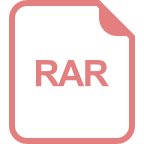
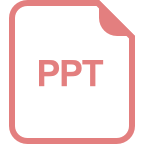
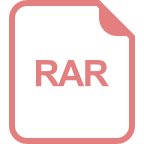
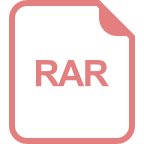
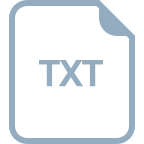
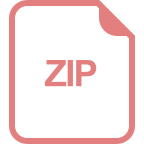
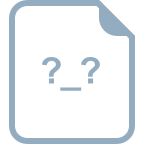
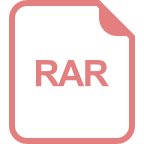
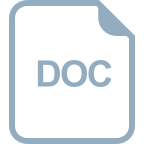
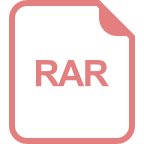