编写遗传算法求解一条高速公路上的充电站选址定容问题
时间: 2023-08-10 10:08:59 浏览: 105
好的,接下来我将详细解释如何编写遗传算法来求解一条高速公路上的充电站选址定容问题。
首先,我们需要定义适应度函数。在这个问题中,适应度函数的计算方式很简单,就是对每个解(即充电站的选址方案)进行评估,计算其总建设成本和总服务成本,并将其累加起来得到适应度值。因此,我们可以定义适应度函数为:
```python
def fitness(solution):
total_construction_cost = 0 # 总建设成本
total_service_cost = 0 # 总服务成本
# 计算每个充电站的建设成本和服务成本
for station in solution:
# 建设成本 = 充电站建设费用 + 联网费用
construction_cost = station[2] + station[3]
# 服务成本 = 充电费用 * 需求量 * 充电效率
service_cost = station[1] * station[4] * station[5]
total_construction_cost += construction_cost
total_service_cost += service_cost
# 计算适应度值
fitness_value = total_construction_cost + total_service_cost
return fitness_value
```
其中,`solution` 是一个列表,表示充电站的选址方案。每个元素是一个元组,包含了充电站的横纵坐标、建设费用、联网费用、充电费用、需求量和充电效率。
接下来,我们需要进行编码。在这个问题中,可以使用二进制编码或者实数编码,本文采用实数编码。具体来说,将每个充电站的选址坐标表示为一个二元组 (x, y),其中 x 和 y 分别为该充电站在高速公路上的横纵坐标。那么一个解就是由多个二元组组成的集合。在实数编码中,我们可以使用一个一维数组来表示这个集合,其中每个元素表示一个二元组的一个坐标值,例如,第 i 个元素表示第 i 个充电站的 x 坐标或者 y 坐标。
因此,我们可以定义编码函数为:
```python
import random
def encode(num_stations, x_min, x_max, y_min, y_max):
solution = []
for i in range(num_stations):
x = random.uniform(x_min, x_max)
y = random.uniform(y_min, y_max)
construction_cost = random.uniform(10, 100) # 建设费用在 10 到 100 之间随机生成
networking_cost = random.uniform(5, 50) # 联网费用在 5 到 50 之间随机生成
charging_fee = random.uniform(0.5, 2) # 充电费用在 0.5 到 2 之间随机生成
demand = random.uniform(100, 1000) # 需求量在 100 到 1000 之间随机生成
efficiency = random.uniform(0.8, 0.95) # 充电效率在 0.8 到 0.95 之间随机生成
station = (x, y, construction_cost, networking_cost, charging_fee, demand, efficiency)
solution.append(station)
return solution
```
其中,`num_stations` 表示充电站数量,`x_min`、`x_max`、`y_min`、`y_max` 表示充电站的横纵坐标范围。
接下来,我们需要使用遗传算法进行优化。遗传算法的主要步骤包括初始化种群、选择、交叉、变异和评估。具体来说,可以按照以下步骤进行:
```python
def genetic_algorithm(num_stations, x_min, x_max, y_min, y_max, pop_size, elite_size, mutation_rate, generations):
# 初始化种群
population = []
for i in range(pop_size):
solution = encode(num_stations, x_min, x_max, y_min, y_max)
population.append(solution)
# 进化
for i in range(generations):
# 评估种群
fitness_scores = []
for solution in population:
fitness_scores.append(fitness(solution))
# 选择优秀的解
elite_index = sorted(range(len(fitness_scores)), key=lambda k: fitness_scores[k])[:elite_size]
elite_solutions = [population[i] for i in elite_index]
# 生成新的种群
new_population = []
for j in range(pop_size):
if j < elite_size: # 保留精英解
new_population.append(elite_solutions[j])
else:
# 选择父代
parent1 = random.choice(elite_solutions)
parent2 = random.choice(elite_solutions)
# 交叉
child = crossover(parent1, parent2)
# 变异
child = mutate(child, mutation_rate)
new_population.append(child)
population = new_population
# 选择最优解
fitness_scores = []
for solution in population:
fitness_scores.append(fitness(solution))
best_index = fitness_scores.index(min(fitness_scores))
best_solution = population[best_index]
return best_solution
```
其中,`num_stations` 表示充电站数量,`x_min`、`x_max`、`y_min`、`y_max` 表示充电站的横纵坐标范围,`pop_size` 表示种群大小,`elite_size` 表示精英解数量,`mutation_rate` 表示变异率,`generations` 表示迭代次数。
在进化过程中,我们使用了轮盘赌选择法来选择父代,并进行了单点交叉和随机变异操作。具体来说,我们可以定义选择函数为:
```python
def selection(fitness_scores):
total_fitness = sum(fitness_scores)
normalized_fitness_scores = [fitness_score / total_fitness for fitness_score in fitness_scores]
cum_sum = 0
for i in range(len(normalized_fitness_scores)):
cum_sum += normalized_fitness_scores[i]
if random.random() < cum_sum:
return i
```
其中,`fitness_scores` 是种群中每个解的适应度值。该函数返回选中的解的索引。
我们可以定义交叉函数为:
```python
def crossover(parent1, parent2):
child = []
for i in range(len(parent1)):
if random.random() < 0.5:
child.append(parent1[i])
else:
child.append(parent2[i])
return child
```
其中,`parent1` 和 `parent2` 分别表示两个父代解,该函数返回一个新的子代解。
我们可以定义变异函数为:
```python
def mutate(solution, mutation_rate):
for i in range(len(solution)):
if random.random() < mutation_rate:
if i % 2 == 0: # 变异 x 坐标
solution[i] = random.uniform(x_min, x_max)
else: # 变异 y 坐标
solution[i] = random.uniform(y_min, y_max)
return solution
```
其中,`solution` 表示一个解,`mutation_rate` 表示变异率。该函数返回一个变异后的新解。
最后,我们可以使用上述函数来求解一条高速公路上的充电站选址定容问题。例如,假设我们需要在 x 轴范围为 [0, 100],y 轴范围为 [0, 50] 的高速公路上选址 5 个充电站,并且需要迭代 100 代,可以使用以下代码:
```python
x_min = 0
x_max = 100
y_min = 0
y_max = 50
num_stations = 5
pop_size = 50
elite_size = 10
mutation_rate = 0.1
generations = 100
best_solution = genetic_algorithm(num_stations, x_min, x_max, y_min, y_max, pop_size, elite_size, mutation_rate, generations)
print(best_solution)
```
该代码将输出最优的选址方案。
阅读全文
相关推荐




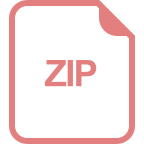









