wxpython+sqlite超市管理系统的全部代码
时间: 2023-12-12 14:02:43 浏览: 37
由于超市管理系统的代码较为复杂,无法在此进行完整的展示,以下是一个简单的 wxpython+sqlite 超市管理系统的部分代码示例,供您参考:
```python
import wx
import sqlite3
class SuperMarket(wx.Frame):
def __init__(self, parent, title):
super(SuperMarket, self).__init__(parent, title=title, size=(800, 600))
self.InitUI()
self.Centre()
self.Show()
def InitUI(self):
# 创建菜单栏
menubar = wx.MenuBar()
fileMenu = wx.Menu()
fileMenu.Append(wx.ID_EXIT, '退出', '退出系统')
menubar.Append(fileMenu, '&文件')
self.SetMenuBar(menubar)
self.Bind(wx.EVT_MENU, self.OnQuit, id=wx.ID_EXIT)
# 创建左侧列表框
self.goods_list = wx.ListCtrl(self, style=wx.LC_REPORT)
self.goods_list.InsertColumn(0, '商品编号', width=100)
self.goods_list.InsertColumn(1, '商品名称', width=150)
self.goods_list.InsertColumn(2, '商品价格', width=100)
self.goods_list.InsertColumn(3, '商品库存', width=100)
self.goods_list.InsertColumn(4, '商品分类', width=100)
self.Bind(wx.EVT_LIST_ITEM_ACTIVATED, self.OnEdit, self.goods_list)
# 创建右侧输入框
self.goods_id = wx.TextCtrl(self)
self.goods_name = wx.TextCtrl(self)
self.goods_price = wx.TextCtrl(self)
self.goods_stock = wx.TextCtrl(self)
self.goods_category = wx.ComboBox(self, choices=['食品', '饮料', '日用品', '化妆品'])
# 创建按钮
self.btn_add = wx.Button(self, label='添加')
self.btn_del = wx.Button(self, label='删除')
self.btn_edit = wx.Button(self, label='编辑')
# 创建布局
hbox1 = wx.BoxSizer(wx.HORIZONTAL)
hbox1.Add(wx.StaticText(self, label='商品编号'), flag=wx.ALIGN_CENTER_VERTICAL)
hbox1.Add(self.goods_id, proportion=1)
hbox2 = wx.BoxSizer(wx.HORIZONTAL)
hbox2.Add(wx.StaticText(self, label='商品名称'), flag=wx.ALIGN_CENTER_VERTICAL)
hbox2.Add(self.goods_name, proportion=1)
hbox3 = wx.BoxSizer(wx.HORIZONTAL)
hbox3.Add(wx.StaticText(self, label='商品价格'), flag=wx.ALIGN_CENTER_VERTICAL)
hbox3.Add(self.goods_price, proportion=1)
hbox4 = wx.BoxSizer(wx.HORIZONTAL)
hbox4.Add(wx.StaticText(self, label='商品库存'), flag=wx.ALIGN_CENTER_VERTICAL)
hbox4.Add(self.goods_stock, proportion=1)
hbox5 = wx.BoxSizer(wx.HORIZONTAL)
hbox5.Add(wx.StaticText(self, label='商品分类'), flag=wx.ALIGN_CENTER_VERTICAL)
hbox5.Add(self.goods_category, proportion=1)
hbox6 = wx.BoxSizer(wx.HORIZONTAL)
hbox6.Add(self.btn_add)
hbox6.Add(self.btn_del)
hbox6.Add(self.btn_edit)
vbox1 = wx.BoxSizer(wx.VERTICAL)
vbox1.Add(self.goods_list, proportion=1, flag=wx.EXPAND|wx.ALL, border=5)
vbox2 = wx.BoxSizer(wx.VERTICAL)
vbox2.Add(hbox1, flag=wx.EXPAND|wx.TOP|wx.LEFT|wx.RIGHT, border=5)
vbox2.Add(hbox2, flag=wx.EXPAND|wx.LEFT|wx.RIGHT, border=5)
vbox2.Add(hbox3, flag=wx.EXPAND|wx.LEFT|wx.RIGHT, border=5)
vbox2.Add(hbox4, flag=wx.EXPAND|wx.LEFT|wx.RIGHT, border=5)
vbox2.Add(hbox5, flag=wx.EXPAND|wx.LEFT|wx.RIGHT, border=5)
vbox2.Add(hbox6, flag=wx.ALIGN_CENTER|wx.BOTTOM|wx.TOP, border=5)
hbox7 = wx.BoxSizer(wx.HORIZONTAL)
hbox7.Add(vbox1, proportion=1, flag=wx.EXPAND|wx.LEFT|wx.RIGHT|wx.TOP|wx.BOTTOM, border=5)
hbox7.Add(vbox2, proportion=0, flag=wx.EXPAND|wx.ALL, border=5)
self.SetSizer(hbox7)
# 连接数据库
self.conn = sqlite3.connect('supermarket.db')
self.cur = self.conn.cursor()
self.cur.execute('''CREATE TABLE IF NOT EXISTS Goods
(id INT PRIMARY KEY NOT NULL,
name TEXT NOT NULL,
price REAL NOT NULL,
stock INT NOT NULL,
category TEXT NOT NULL)''')
self.conn.commit()
def OnQuit(self, e):
self.Close()
def OnEdit(self, e):
index = e.GetIndex()
item = self.goods_list.GetItem(index, 0)
self.goods_id.SetValue(item.GetText())
item = self.goods_list.GetItem(index, 1)
self.goods_name.SetValue(item.GetText())
item = self.goods_list.GetItem(index, 2)
self.goods_price.SetValue(item.GetText())
item = self.goods_list.GetItem(index, 3)
self.goods_stock.SetValue(item.GetText())
item = self.goods_list.GetItem(index, 4)
self.goods_category.SetValue(item.GetText())
def LoadData(self):
self.goods_list.DeleteAllItems()
self.cur.execute('SELECT * FROM Goods')
rows = self.cur.fetchall()
for row in rows:
index = self.goods_list.InsertItem(0, str(row[0]))
self.goods_list.SetItem(index, 1, row[1])
self.goods_list.SetItem(index, 2, str(row[2]))
self.goods_list.SetItem(index, 3, str(row[3]))
self.goods_list.SetItem(index, 4, row[4])
def OnAdd(self, e):
id = self.goods_id.GetValue()
name = self.goods_name.GetValue()
price = self.goods_price.GetValue()
stock = self.goods_stock.GetValue()
category = self.goods_category.GetValue()
if id and name and price and stock and category:
self.cur.execute('INSERT INTO Goods VALUES (?, ?, ?, ?, ?)', (id, name, price, stock, category))
self.conn.commit()
self.LoadData()
else:
wx.MessageBox('请填写完整信息!', '错误', wx.OK|wx.ICON_ERROR)
def OnDel(self, e):
id = self.goods_id.GetValue()
if id:
self.cur.execute('DELETE FROM Goods WHERE id = ?', (id,))
self.conn.commit()
self.LoadData()
def OnEdit(self, e):
id = self.goods_id.GetValue()
name = self.goods_name.GetValue()
price = self.goods_price.GetValue()
stock = self.goods_stock.GetValue()
category = self.goods_category.GetValue()
if id and name and price and stock and category:
self.cur.execute('UPDATE Goods SET name = ?, price = ?, stock = ?, category = ? WHERE id = ?', (name, price, stock, category, id))
self.conn.commit()
self.LoadData()
else:
wx.MessageBox('请填写完整信息!', '错误', wx.OK|wx.ICON_ERROR)
if __name__ == '__main__':
app = wx.App()
SuperMarket(None, title='超市管理系统')
app.MainLoop()
```
此代码仅为示例,实际情况可能存在差异,仅供参考。
相关推荐
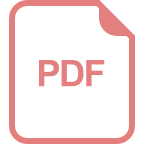
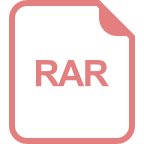
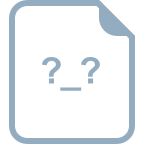














