QT reference to non-static member function must be
时间: 2024-05-13 16:18:35 浏览: 281
This error message usually occurs when you try to pass a non-static member function as a callback function to a Qt signal.
To solve this issue, you need to ensure that the member function is declared as `static` or that it is a non-member function. If you cannot declare the member function as static, you can use a lambda function or a `std::bind` expression to wrap the member function into a callable object that can be passed as a callback.
For example, suppose you have a class `MyClass` with a non-static member function `myFunction`, and you want to connect a signal to this function:
```cpp
class MyClass : public QObject
{
Q_OBJECT
public:
void myFunction(int value);
};
MyClass myObject;
connect(mySignal, &MySignal::valueChanged, &myObject, &MyClass::myFunction); // Error!
```
Since `myFunction` is not static, you will get the error message "reference to non-static member function must be called" when you try to compile this code. To solve this, you can wrap `myFunction` into a lambda function:
```cpp
class MyClass : public QObject
{
Q_OBJECT
public:
void myFunction(int value);
};
MyClass myObject;
connect(mySignal, &MySignal::valueChanged, &myObject,
[](int value){ myObject.myFunction(value); }); // OK!
```
Alternatively, you can use `std::bind`:
```cpp
class MyClass : public QObject
{
Q_OBJECT
public:
void myFunction(int value);
};
MyClass myObject;
connect(mySignal, &MySignal::valueChanged, &myObject,
std::bind(&MyClass::myFunction, &myObject, std::placeholders::_1)); // OK!
```
Both of these solutions create a callable object that calls `myFunction` on `myObject` with the correct parameter.
阅读全文
相关推荐
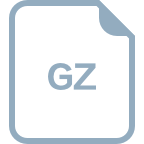


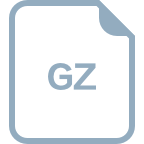
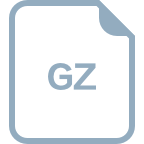