代码实现生产者消费者问题
时间: 2024-04-26 21:05:16 浏览: 9
生产者消费者问题是一个经典的同步问题,主要涉及到生产者和消费者两种角色,生产者生产数据,消费者消费数据,两者必须同步进行,避免生产者生产过多数据造成浪费,或者消费者消费过多数据造成程序崩溃等问题。
下面是一个简单的 Python 代码实现:
``` python
import threading
import time
import random
queue = []
MAX_NUM = 10
condition = threading.Condition()
class ProducerThread(threading.Thread):
def run(self):
nums = range(5)
global queue
while True:
condition.acquire()
if len(queue) == MAX_NUM:
print("Queue full, producer is waiting")
condition.wait()
print("Space in queue, Consumer notified the producer")
num = random.choice(nums)
queue.append(num)
print("Produced", num)
condition.notify()
condition.release()
time.sleep(random.random())
class ConsumerThread(threading.Thread):
def run(self):
global queue
while True:
condition.acquire()
if not queue:
print("Nothing in queue, consumer is waiting")
condition.wait()
print("Producer added something to queue and notified the consumer")
num = queue.pop(0)
print("Consumed", num)
condition.notify()
condition.release()
time.sleep(random.random())
ProducerThread().start()
ConsumerThread().start()
```
在这个例子中,我们定义了一个队列 `queue`,最大长度为 `MAX_NUM`,用 `condition` 实现同步。生产者线程 `ProducerThread` 生产数据,消费者线程 `ConsumerThread` 消费数据,它们都会在队列长度达到最大值或者队列为空时等待,直到被唤醒。
我们可以通过调整 `MAX_NUM` 和线程的数量,来模拟不同的场景和效果。
相关推荐
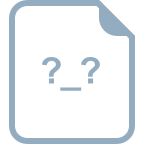














