FileSystemResource oom
时间: 2023-12-14 17:34:12 浏览: 32
FileSystemResource是Spring框架中的一个类,用于加载文件资源。如果使用不当,可能会导致内存溢出(OOM)的问题。具体来说,如果使用FileSystemResource加载一个过大的文件,会导致内存溢出。因为FileSystemResource会将整个文件读入内存中,如果文件过大,就会导致内存溢出。
为了避免FileSystemResource OOM的问题,可以采用以下两种方法:
1.使用InputStreamResource代替FileSystemResource,InputStreamResource可以流式地读取文件,不会将整个文件读入内存中。
2.对于较大的文件,可以使用RandomAccessFile进行分块读取,避免一次性将整个文件读入内存中。
下面是两种解决方法的示例代码:
1.使用InputStreamResource代替FileSystemResource
```java
@GetMapping("/download")
public ResponseEntity<InputStreamResource> downloadFile() throws IOException {
File file = new File("path/to/file");
InputStreamResource resource = new InputStreamResource(new FileInputStream(file));
HttpHeaders headers = new HttpHeaders();
headers.add("Content-Disposition", "attachment; filename=" + file.getName());
return ResponseEntity.ok()
.headers(headers)
.contentLength(file.length())
.contentType(MediaType.parseMediaType("application/octet-stream"))
.body(resource);
}
```
2.使用RandomAccessFile进行分块读取
```java
@GetMapping("/download")
public ResponseEntity<InputStreamResource> downloadFile() throws IOException {
File file = new File("path/to/file");
RandomAccessFile raf = new RandomAccessFile(file, "r");
long contentLength = raf.length();
HttpHeaders headers = new HttpHeaders();
headers.add("Content-Disposition", "attachment; filename=" + file.getName());
InputStreamResource resource = new InputStreamResource(new FileInputStream(file)) {
@Override
public long contentLength() throws IOException {
return contentLength;
}
@Override
public InputStream getInputStream() throws IOException {
return new FileInputStream(file) {
@Override
public int available() throws IOException {
long available = contentLength - raf.getFilePointer();
return available > Integer.MAX_VALUE ? Integer.MAX_VALUE : (int) available;
}
@Override
public int read(byte[] b, int off, int len) throws IOException {
raf.readFully(b, off, len);
return len;
}
};
}
};
return ResponseEntity.ok()
.headers(headers)
.contentLength(contentLength)
.contentType(MediaType.parseMediaType("application/octet-stream"))
.body(resource);}
```
相关推荐
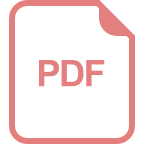
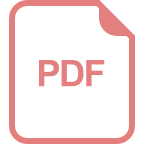
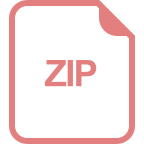














