提交按钮不能设置宽高吗
时间: 2024-05-05 21:22:01 浏览: 14
可以设置宽高,可以使用CSS的width和height属性来设置提交按钮的宽高。例如:
```html
<button type="submit" style="width: 100px; height: 50px;">提交</button>
```
也可以将样式写在CSS样式表中,然后通过类名或ID来应用样式。例如:
```html
<button type="submit" class="submit-btn">提交</button>
```
```css
.submit-btn {
width: 100px;
height: 50px;
}
```
相关问题
php使用表单上传图片,并提交缩略图最大宽高,并保持文件夹,然后使用缩略图函数生成缩略图并输出原图和缩略图
好的,我来回答你的问题。首先,你可以使用 HTML 表单和 PHP 来上传图片。以下是实现上传图片的 PHP 代码:
```php
<?php
if(isset($_POST['submit'])){
$target_dir = "uploads/";
$target_file = $target_dir . basename($_FILES["fileToUpload"]["name"]);
$imageFileType = strtolower(pathinfo($target_file,PATHINFO_EXTENSION));
$max_width = $_POST['max_width'];
$max_height = $_POST['max_height'];
$check = getimagesize($_FILES["fileToUpload"]["tmp_name"]);
if($check !== false){
if($imageFileType == "jpg" || $imageFileType == "png" || $imageFileType == "jpeg" || $imageFileType == "gif" ){
if(move_uploaded_file($_FILES["fileToUpload"]["tmp_name"], $target_file)){
$image = $target_dir . basename($_FILES["fileToUpload"]["name"]);
$thumbnail = $target_dir . 'thumbnail_' . basename($_FILES["fileToUpload"]["name"]);
list($width, $height) = getimagesize($image);
$ratio = min($max_width/$width, $max_height/$height);
$new_width = $width * $ratio;
$new_height = $height * $ratio;
$thumb = imagecreatetruecolor($new_width, $new_height);
if($imageFileType == "jpg" || $imageFileType == "jpeg"){
$source = imagecreatefromjpeg($image);
}else if($imageFileType == "png"){
$source = imagecreatefrompng($image);
}else if($imageFileType == "gif"){
$source = imagecreatefromgif($image);
}
imagecopyresampled($thumb, $source, 0, 0, 0, 0, $new_width, $new_height, $width, $height);
if($imageFileType == "jpg" || $imageFileType == "jpeg"){
imagejpeg($thumb, $thumbnail);
}else if($imageFileType == "png"){
imagepng($thumb, $thumbnail);
}else if($imageFileType == "gif"){
imagegif($thumb, $thumbnail);
}
echo "The original image is <img src='$image'/><br>";
echo "The thumbnail image is <img src='$thumbnail'/>";
}else{
echo "Sorry, there was an error uploading your file.";
}
}else{
echo "Sorry, only JPG, JPEG, PNG & GIF files are allowed.";
}
}else{
echo "File is not an image.";
}
}
?>
```
这个 PHP 代码实现了上传图片并生成缩略图的功能,其中包括:
- 检查上传的文件是否是一个图像文件
- 检查上传的文件是否已经存在
- 检查上传的文件类型是否是允许的类型
- 移动上传的文件到指定文件夹
- 计算缩略图的大小
- 创建缩略图
- 输出原图和缩略图
你可以把上面的 PHP 代码放在一个 HTML 表单页面中,这样就可以通过浏览器上传图片并生成缩略图了。以下是一个简单的 HTML 表单页面:
```html
<!DOCTYPE html>
<html>
<head>
<title>Upload Image and Generate Thumbnail</title>
</head>
<body>
<form action="upload.php" method="post" enctype="multipart/form-data">
Select image to upload:
<input type="file" name="fileToUpload" id="fileToUpload"><br><br>
Max Width of Thumbnail:
<input type="number" name="max_width" value="200"><br><br>
Max Height of Thumbnail:
<input type="number" name="max_height" value="200"><br><br>
<input type="submit" value="Upload Image and Generate Thumbnail" name="submit">
</form>
</body>
</html>
```
在这个 HTML 表单页面中,你可以选择一个图片文件,设置缩略图的最大宽度和最大高度,然后点击提交按钮来上传图片并生成缩略图。
我希望这个代码对你有所帮助!
1. 基于JSP+Servlet+JavaBean的设计模式,设计一个Web应用程序,当用户在提交页面上输入矩形的长和宽,提交后显示出矩形的周长和面积。
首先,我们需要创建一个 JSP 页面,用于用户输入矩形的长和宽,并提交这些值。页面的代码如下:
```
<html>
<head>
<title>计算矩形周长和面积</title>
</head>
<body>
<h1>计算矩形周长和面积</h1>
<form action="RectangleServlet" method="post">
<label>长:</label><input type="text" name="length"/><br>
<label>宽:</label><input type="text" name="width"/><br>
<input type="submit" value="提交"/>
</form>
</body>
</html>
```
在表单中,我们定义了两个输入框和一个提交按钮,并将表单的提交地址设置为“RectangleServlet”。
然后,我们需要创建一个 Servlet 类,用于处理表单提交的数据,计算矩形的周长和面积,并将结果返回给用户。Servlet 类的代码如下:
```
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class RectangleServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 获取用户输入的长和宽
double length = Double.parseDouble(request.getParameter("length"));
double width = Double.parseDouble(request.getParameter("width"));
// 计算周长和面积
double perimeter = 2 * (length + width);
double area = length * width;
// 将结果返回给用户
response.setContentType("text/html;charset=UTF-8");
response.getWriter().println("<html>");
response.getWriter().println("<head><title>矩形计算结果</title></head>");
response.getWriter().println("<body>");
response.getWriter().println("<h1>矩形计算结果</h1>");
response.getWriter().println("<p>长:" + length + "</p>");
response.getWriter().println("<p>宽:" + width + "</p>");
response.getWriter().println("<p>周长:" + perimeter + "</p>");
response.getWriter().println("<p>面积:" + area + "</p>");
response.getWriter().println("</body>");
response.getWriter().println("</html>");
}
}
```
在 doPost 方法中,我们首先获取用户输入的长和宽,并进行类型转换。然后,我们计算矩形的周长和面积,并将计算结果返回给用户。
最后,我们需要将 Servlet 类部署到 Web 服务器上,并将 JSP 页面和 Servlet 类进行关联。具体步骤可以参考各种 Web 服务器的部署文档。部署完成后,用户可以访问 JSP 页面,输入矩形的长和宽,提交后即可查看矩形的周长和面积。
相关推荐
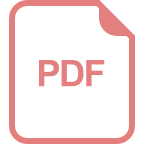
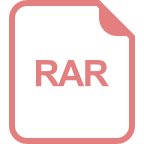










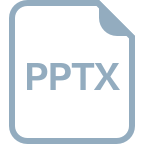