将三名学生的学号、姓名、班级信息、3门课程成绩、平均成绩,输出到一个f1文件中。将这3个学生按照平均成绩从高到低排序后,输出到f2文件中
时间: 2024-06-08 18:09:22 浏览: 3
下面是一个Python实现的示例代码,供参考:
```python
# 定义学生类
class Student:
def __init__(self, id, name, cls, score1, score2, score3):
self.id = id
self.name = name
self.cls = cls
self.score1 = score1
self.score2 = score2
self.score3 = score3
self.avg_score = (score1 + score2 + score3) / 3
# 创建三个学生对象
stu1 = Student("001", "张三", "一班", 85, 90, 92)
stu2 = Student("002", "李四", "一班", 91, 89, 87)
stu3 = Student("003", "王五", "一班", 78, 86, 92)
# 将学生信息写入f1文件
with open("f1", "w") as f1:
for stu in [stu1, stu2, stu3]:
f1.write(stu.id + " " + stu.name + " " + stu.cls + " ")
f1.write(str(stu.score1) + " " + str(stu.score2) + " " + str(stu.score3) + " ")
f1.write(str(stu.avg_score) + "\n")
# 按照平均成绩从高到低排序
students = [stu1, stu2, stu3]
students.sort(key=lambda x: x.avg_score, reverse=True)
# 将排序后的学生信息写入f2文件
with open("f2", "w") as f2:
for stu in students:
f2.write(stu.id + " " + stu.name + " " + stu.cls + " ")
f2.write(str(stu.score1) + " " + str(stu.score2) + " " + str(stu.score3) + " ")
f2.write(str(stu.avg_score) + "\n")
```
以上代码会生成两个文件:f1 和 f2。其中,f1 文件包含所有学生的信息,f2 文件按照平均成绩从高到低排序后输出学生信息。您可以根据需要修改输出的文件名和路径。
相关推荐
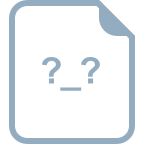
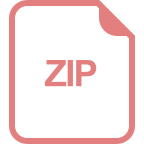
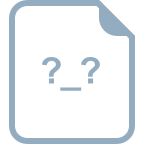















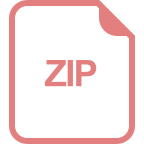