python打印数据用tkinter库显示
时间: 2024-02-17 16:32:15 浏览: 117
使用 Tkinter 库,你可以创建一个 GUI 界面并在其中显示数据。下面是一个简单的示例代码,它读取一个文件中的数据,并将其显示在一个文本框中:
```python
import tkinter as tk
# 创建窗口
root = tk.Tk()
root.title("数据展示")
# 创建文本框
text = tk.Text(root)
text.pack()
# 读取数据
with open("data.txt", "r") as file:
data = file.read()
# 在文本框中显示数据
text.insert(tk.END, data)
# 运行窗口
root.mainloop()
```
在上面的代码中,我们首先创建了一个窗口,然后创建了一个文本框,将其打包并显示在窗口中。接下来,我们使用 `open` 函数读取一个文件中的数据,并将其存储在 `data` 变量中。最后,我们使用 `text.insert` 方法将数据插入到文本框中,并使用 `mainloop` 方法运行窗口。
相关问题
python实现将输入框内的数据进行RSA加密,再打印在tkinter文本框中
下面是一个简单的示例程序,使用Python的RSA库进行加密:
```python
from tkinter import *
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
# 生成RSA密钥对
key = RSA.generate(2048)
pub_key = key.publickey()
# 创建加密器
cipher = PKCS1_OAEP.new(pub_key)
def encrypt():
# 获取输入框内容
data = entry.get()
# 使用加密器进行加密
encrypted_data = cipher.encrypt(data.encode())
# 将加密后的内容打印到文本框中
text.insert(END, encrypted_data.hex() + '\n')
# 创建窗口和控件
root = Tk()
entry = Entry(root)
entry.pack()
button = Button(root, text='Encrypt', command=encrypt)
button.pack()
text = Text(root)
text.pack()
root.mainloop()
```
在上面的示例程序中,我们使用`Crypto`库生成RSA密钥对,并使用`PKCS1_OAEP`算法创建加密器。在`encrypt`函数中,我们获取输入框内容,使用加密器进行加密,并将加密后的内容打印到文本框中。
python实现将输入框内的数据进行md5加密,再打印在tkinter文本框中
可以使用Python内置的hashlib模块来实现MD5加密,然后使用Tkinter的Text组件来显示加密后的结果。以下是一个简单的示例:
```python
import hashlib
import tkinter as tk
def md5_encrypt():
# 获取输入框中的文本并进行MD5加密
plaintext = input_box.get()
ciphertext = hashlib.md5(plaintext.encode()).hexdigest()
# 在文本框中显示加密后的结果
output_box.insert('end', ciphertext + '\n')
# 创建主窗口和输入输出文本框
root = tk.Tk()
input_box = tk.Entry(root)
output_box = tk.Text(root)
# 创建按钮并绑定事件处理函数
encrypt_button = tk.Button(root, text='Encrypt', command=md5_encrypt)
# 将控件放置到主窗口中
input_box.pack()
encrypt_button.pack()
output_box.pack()
root.mainloop()
```
在上述代码中,我们创建了一个输入框(Entry)、一个文本框(Text)和一个按钮(Button)。当用户在输入框中输入文本并点击按钮时,程序会将输入文本进行MD5加密,并将加密结果显示在文本框中。注意,我们使用了Text组件的`insert()`方法来将文本添加到文本框中。`'end'`参数表示将文本添加到文本框的末尾。
阅读全文
相关推荐
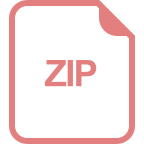
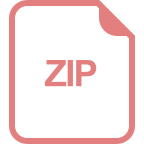
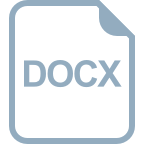
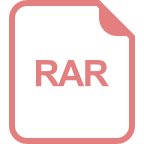
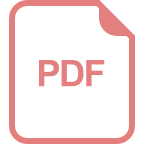
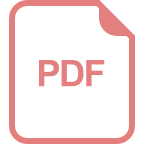
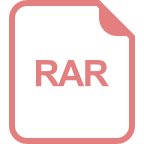
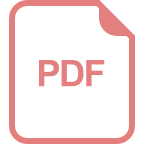
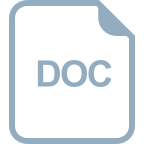
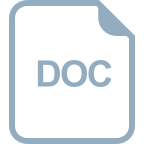
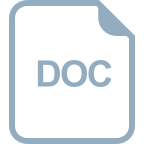
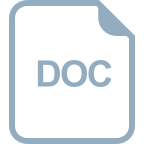


