#include <stdio.h> #include <stdlib.h> static char buff[256]; static char* string; int main() { printf("Please input your string:"); gets(string); printf("\nYour string is:%s\n",string); }
时间: 2024-01-17 16:04:39 浏览: 75
这段代码是一个简单的C程序,它的功能是从标准输入中读取一行字符串并输出。
然而,这段代码存在一个严重的问题:它使用了不安全的gets函数来读取输入,可能会导致缓冲区溢出漏洞。正确的做法是使用fgets函数来代替gets函数,限制读取的字符数量。
另外,这段代码声明了一个静态的字符数组buff,但是却没有使用它。而指针变量string却没有被初始化,使用时会导致未定义的行为。应该将string指向buff数组,或者使用动态内存分配函数malloc来为string分配内存。
改进后的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_LEN 256
int main() {
char buff[MAX_LEN];
char *string = (char*)malloc(MAX_LEN * sizeof(char));
printf("Please input your string:");
fgets(buff, MAX_LEN, stdin);
sscanf(buff, "%s", string); // 去掉字符串末尾的换行符
printf("\nYour string is:%s\n", string);
free(string);
return 0;
}
```
相关问题
2.使用gdb或者是eclipse调试工具调试程序错误,代码如下: #include <stdio.h> #include <stdlib.h> static char buff[256]; static char* string; int main() { printf("Please input your string:"); gets(string); printf("\nYour string is:%s\n",string); }
好的,这是一个非常简单的C程序,但是有一个明显的错误,就是没有为指针变量 `string` 分配内存空间,这会导致在调用 `gets()` 函数时发生未定义的行为,可能会导致程序崩溃或者出现意想不到的结果。
修改代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
static char buff[256];
static char* string;
int main() {
printf("Please input your string:");
fgets(buff, sizeof(buff), stdin);
string = strdup(buff);
printf("\nYour string is:%s\n", string);
free(string);
return 0;
}
```
在这个版本的代码中,我们使用了 `fgets()` 函数来读取用户输入的字符串,这个函数比 `gets()` 更加安全,因为它可以指定读取字符串的最大长度,防止缓冲区溢出。然后,我们使用 `strdup()` 函数来为 `string` 分配内存空间,并将输入的字符串复制到这个新的内存空间中。最后,需要释放内存空间,以免内存泄漏。
关于调试工具,我推荐使用 `gdb` 进行单步调试,在命令行中输入 `gdb [可执行文件名]` 即可启动 `gdb`,然后使用 `run` 命令运行程序,在程序出现错误时使用 `backtrace` 命令查看函数调用栈,使用 `print` 命令查看变量的值,使用 `step` 命令逐行执行代码,帮助你找到错误的源头。
#include<stdio.h>#include<stdlib.h>#include<string.h>
在C语言中,`#include<stdio.h>`、`#include<stdlib.h>`和`#include<string.h>`分别包含了输入/输出、内存管理以及字符串处理相关的库。这里给你演示如何使用这些头文件中的函数:
1. **输入/输出(stdio.h)**:
```c
#include<stdio.h>
int main() {
printf("Hello, World!\n"); // 使用printf输出字符串
scanf("%s", "Enter your name: "); // 使用scanf从用户那里读取输入
return 0;
}
```
2. **内存管理(stdlib.h)**:
```c
#include<stdlib.h>
int *createArray(int size) {
int *array = malloc(size * sizeof(int)); // 分配动态内存
return array;
}
void freeMemory(int *array, int size) {
free(array); // 释放已分配的内存
}
```
3. **字符串处理(string.h)**:
```c
#include<string.h>
char* copyString(const char* src) {
char* dest = (char*)malloc(strlen(src) + 1); // 动态分配内存复制字符串
strcpy(dest, src);
return dest;
}
```
注意,C语言的标准库函数可能需要链接到适当的库,如`-lstdc++` 或 `-lm`,具体取决于编译器设置。
阅读全文
相关推荐
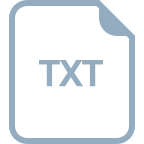
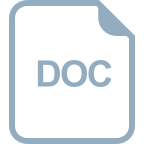
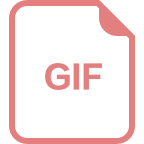
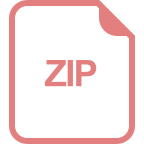
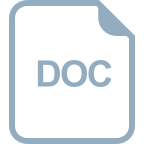
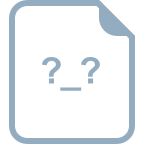
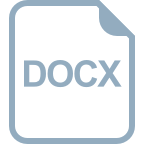

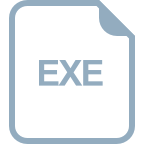
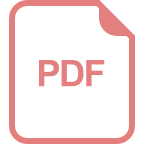
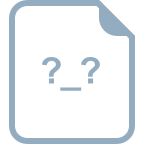
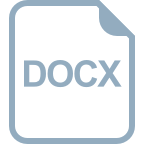
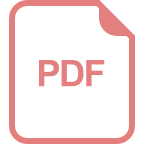
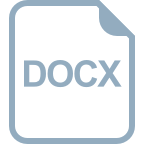
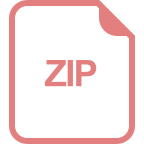