thinker-md实现代码
时间: 2024-05-13 18:14:24 浏览: 126
以下是使用 Python 实现的 thinker-md 的代码示例:
```python
import os
import re
# 读取文件内容
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
content = f.read()
return content
# 将 Markdown 转换为 HTML
def markdown_to_html(content):
# 标题
content = re.sub(r'^#\s+(.*)$', r'<h1>\1</h1>', content, flags=re.M)
content = re.sub(r'^##\s+(.*)$', r'<h2>\1</h2>', content, flags=re.M)
content = re.sub(r'^###\s+(.*)$', r'<h3>\1</h3>', content, flags=re.M)
content = re.sub(r'^####\s+(.*)$', r'<h4>\1</h4>', content, flags=re.M)
content = re.sub(r'^#####\s+(.*)$', r'<h5>\1</h5>', content, flags=re.M)
content = re.sub(r'^######\s+(.*)$', r'<h6>\1</h6>', content, flags=re.M)
# 强调
content = re.sub(r'\*\*(.*)\*\*', r'<strong>\1</strong>', content)
content = re.sub(r'__(.*)__', r'<strong>\1</strong>', content)
content = re.sub(r'\*(.*)\*', r'<em>\1</em>', content)
content = re.sub(r'_(.*)_', r'<em>\1</em>', content)
# 列表
content = re.sub(r'^\*\s+(.*)$', r'<li>\1</li>', content, flags=re.M)
content = re.sub(r'<li>(.*)</li>', r'<ul><li>\1</li></ul>', content, flags=re.S)
# 代码块
content = re.sub(r'```(.*?)```', r'<pre><code>\1</code></pre>', content, flags=re.S)
# 链接
content = re.sub(r'\[(.*?)\]\((.*?)\)', r'<a href="\2">\1</a>', content)
return content
if __name__ == '__main__':
file_path = 'example.md'
content = read_file(file_path)
html_content = markdown_to_html(content)
print(html_content)
```
该代码实现了以下功能:
1. 读取文件内容;
2. 将 Markdown 中的标题、强调、列表、代码块和链接转换为 HTML 标记;
3. 输出转换后的 HTML 内容。
你可以将代码保存为 `thinker_md.py` 文件,并在命令行中运行 `python thinker_md.py` 来执行代码并输出 HTML 内容。
阅读全文
相关推荐
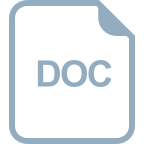
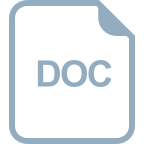
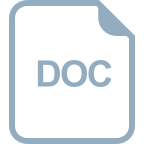
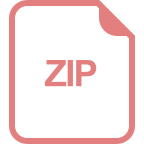
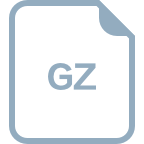
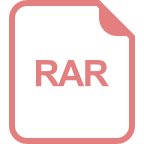
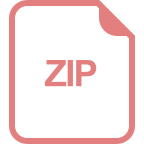
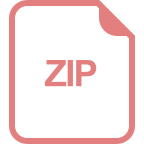
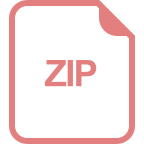
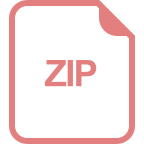
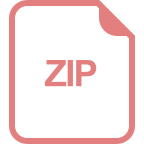
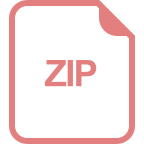
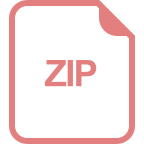
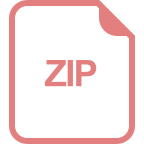
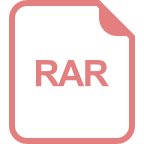
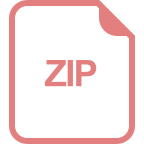
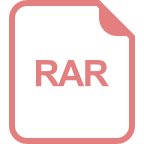
