Polycarp doesn't like integers that are divisible by 3 or end with the digit 3 in their decimal representation. Integers that meet both conditions are disliked by Polycarp, too. Polycarp starts to write out the positive (greater than 0 ) integers which he likes: 1,2,4,5,7,8,10,11,14,16,… . Output the k -th element of this sequence (the elements are numbered from 1 ). Input The first line contains one integer t (1≤t≤100 ) — the number of test cases. Then t test cases follow. Each test case consists of one line containing one integer k (1≤k≤1000 ). Output For each test case, output in a separate line one integer x — the k -th element of the sequence that was written out by Polycarp.
时间: 2024-01-05 12:02:48 浏览: 110
Sure, I understand your question. Based on the given conditions, Polycarp likes positive integers that are not divisible by 3 and do not end with the digit 3. We can generate this sequence of numbers as follows:
1, 2, 4, 5, 7, 8, 10, 11, 14, 16, 17, 19, 20, 22, 25, 28, 29, 40, 44, 46, 50, 52, 55, 58, 59, 70, 71, 74, 76, 79, 80, 82, 85, 88, 91, 94, 95, 97, 98, 100, 101, 104, 106, 107, 110, 112, 115, 118, 119, 121, ...
To find the k-th element of this sequence, we can simply iterate through the sequence and stop at the k-th element. Here's the code to do that:
```
#include <iostream>
#include <vector>
using namespace std;
bool is_disliked(int n) {
return (n % 3 == 0) || (n % 10 == 3);
}
vector<int> generate_sequence(int n) {
vector<int> seq;
int i = 1;
while (seq.size() < n) {
if (!is_disliked(i)) {
seq.push_back(i);
}
i++;
}
return seq;
}
int main() {
int t;
cin >> t;
while (t--) {
int k;
cin >> k;
vector<int> seq = generate_sequence(k);
cout << seq[k-1] << endl;
}
return 0;
}
```
The `is_disliked()` function checks if a number is disliked by Polycarp based on the given conditions. The `generate_sequence()` function generates the sequence of liked numbers by iterating through all positive integers and adding them to the sequence if they are not disliked.
In the main function, we first read in the number of test cases `t`. Then we loop `t` times and for each test case, we read in the value of `k`, generate the sequence of liked numbers of length `k`, and output the k-th element of that sequence.
阅读全文
相关推荐
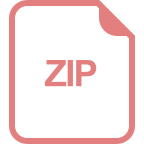

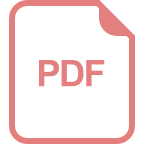
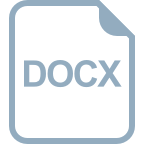
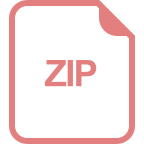
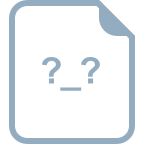
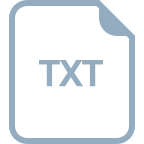
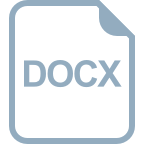
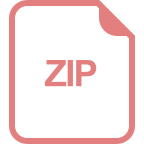
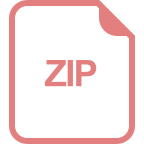
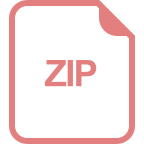
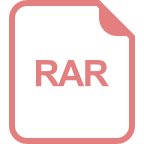
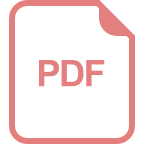
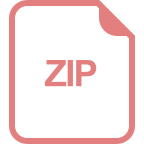