java 代码2. 使用Spring security实现用户认证和授权的项目: (1)数据准备,在第三章实验3的基础上添加表t_customer、t_authoytity、t_customer_authority,添加若干登录用户和角色的实验数据。 (2)SpringBoot自动创建spring web项目,选取Spring web依赖、Thymeleaf、Jpa、mysqldriver依赖。 (3)在pojo包中编写实体类Customer和Authority实体类,并按照jpa的映射方式进行注解。 (4)在repository包中创建CustomerRepository和AuthorityRepository接口,创建并装配findByUsername和findAuthoritiesByUsername方法。 (5)在service包中创建CustomerService类实现getCustomer()和getCustomerAuthority()方法,并结合RedisApi对用户和权限数据实施redis缓存;创建UserDetailServiceImpl类,实现加载用户详细信息UserDetails 的loadUserByUsername()方法。。 (6)在controller包中创建FilmController类实现@GetMapping("/detail/{type}/{path}")请求映射以及对应的方法。 (7)在pom文件中引入spring security和jpa、redis依赖
时间: 2024-03-17 16:44:04 浏览: 79
很抱歉,我无法提供具体的代码,因为这是一个比较复杂的项目,需要您根据具体的业务需求和数据结构进行编写。但是,以下是一些关于使用Spring Security实现用户认证和授权的项目的代码实现的参考:
1. 实体类:
```
@Entity
@Table(name = "t_customer")
public class Customer implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String username;
@Column(nullable = false)
private String password;
@ManyToMany(fetch = FetchType.EAGER)
@JoinTable(name = "t_customer_authority", joinColumns = {@JoinColumn(name = "customer_id", referencedColumnName = "id")},
inverseJoinColumns = {@JoinColumn(name = "authority_id", referencedColumnName = "id")})
private Set<Authority> authorities;
// getters and setters
}
@Entity
@Table(name = "t_authority")
public class Authority implements Serializable {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false, unique = true)
private String name;
@ManyToMany(mappedBy = "authorities")
private Set<Customer> customers;
// getters and setters
}
```
2. Repository接口:
```
public interface CustomerRepository extends JpaRepository<Customer, Long> {
Customer findByUsername(String username);
}
public interface AuthorityRepository extends JpaRepository<Authority, Long> {
Set<Authority> findAuthoritiesByUsername(String username);
}
```
3. Service类:
```
@Service
public class CustomerService {
@Autowired
private CustomerRepository customerRepository;
@Autowired
private RedisApi redisApi;
public Customer getCustomer(String username) {
Customer customer = redisApi.get("customer:" + username, Customer.class);
if (customer == null) {
customer = customerRepository.findByUsername(username);
redisApi.set("customer:" + username, customer, 60 * 60 * 24);
}
return customer;
}
public Set<Authority> getCustomerAuthority(String username) {
Set<Authority> authorities = redisApi.get("authorities:" + username, new TypeReference<Set<Authority>>() {});
if (authorities == null) {
authorities = authorityRepository.findAuthoritiesByUsername(username);
redisApi.set("authorities:" + username, authorities, 60 * 60 * 24);
}
return authorities;
}
}
@Service
public class UserDetailServiceImpl implements UserDetailsService {
@Autowired
private CustomerService customerService;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
Customer customer = customerService.getCustomer(username);
if (customer == null) {
throw new UsernameNotFoundException("用户名不存在!");
}
Set<Authority> authorities = customerService.getCustomerAuthority(username);
List<GrantedAuthority> grantedAuthorities = new ArrayList<>();
for (Authority authority : authorities) {
grantedAuthorities.add(new SimpleGrantedAuthority(authority.getName()));
}
return new User(customer.getUsername(), customer.getPassword(), grantedAuthorities);
}
}
```
4. Controller类:
```
@Controller
public class FilmController {
@GetMapping("/detail/{type}/{path}")
public String detail(@PathVariable("type") String type, @PathVariable("path") String path) {
// 处理请求
return "detail";
}
}
```
5. pom.xml文件:
```
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-config</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-web</artifactId>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
</dependency>
</dependencies>
```
以上是一个简单的使用Spring Security实现用户认证和授权的项目的代码实现参考,希望能对您有所帮助。
阅读全文
相关推荐
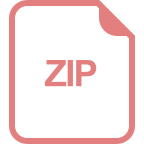
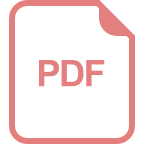
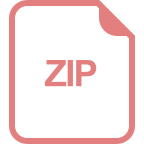
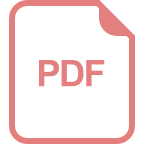
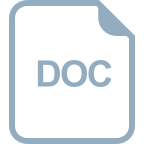
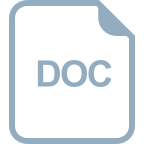
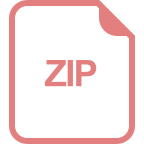
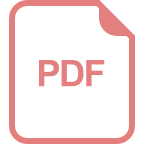
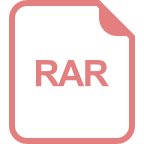
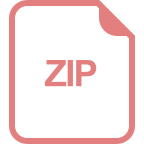
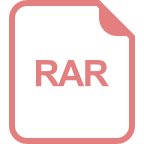
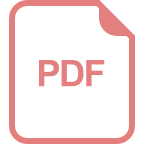
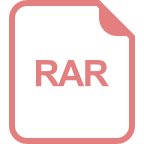
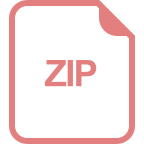
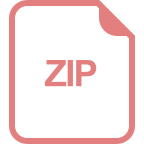
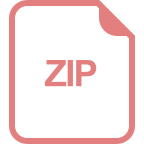
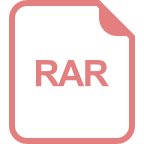
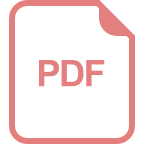