生成光立方显示数字的代码
时间: 2024-02-12 10:07:45 浏览: 206
以下是一个简单的 Python 代码示例,使用 Pygame 库生成一个光立方,并在其中显示数字:
```python
import pygame
import time
# 初始化 Pygame
pygame.init()
# 设置立方体大小和位置
CUBE_SIZE = 100
CUBE_POS = (200, 200)
# 设置窗口大小和标题
WINDOW_SIZE = (600, 400)
WINDOW_TITLE = '光立方显示数字'
screen = pygame.display.set_mode(WINDOW_SIZE)
pygame.display.set_caption(WINDOW_TITLE)
# 设置颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
# 创建字体对象
font = pygame.font.Font(None, 60)
# 定义数字列表
numbers = [
'111101101101111', # 0
'001001001001001', # 1
'111001111100111', # 2
'111001111001111', # 3
'101101111001001', # 4
'111100111001111', # 5
'111100111101111', # 6
'111001001001001', # 7
'111101111101111', # 8
'111101111001111', # 9
]
# 定义函数,绘制立方体的一个面
def draw_face(surface, color, pos, size, on):
x, y = pos
w, h = size
if on:
pygame.draw.rect(surface, color, (x, y, w, h))
else:
pygame.draw.rect(surface, BLACK, (x, y, w, h))
# 定义函数,绘制光立方
def draw_cube(surface, pos, size, number):
x, y = pos
w, h = size
for i, row in enumerate(number):
for j, col in enumerate(row):
# 计算每个面的位置和大小
face_pos = (x + j * w, y + i * h)
face_size = (w, h)
# 根据数字中的 1 或 0,决定是否绘制面
if col == '1':
draw_face(surface, RED, face_pos, face_size, True)
else:
draw_face(surface, WHITE, face_pos, face_size, False)
# 循环显示数字
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 获取当前时间的秒数,并转换为两位数字的字符串
seconds = time.strftime('%S')
if seconds[0] == '0':
seconds = seconds[1]
# 根据字符串中的数字,获取数字对应的列表
number = numbers[int(seconds)]
# 清空屏幕
screen.fill(BLACK)
# 绘制光立方
draw_cube(screen, CUBE_POS, (CUBE_SIZE, CUBE_SIZE), number)
# 绘制数字文本
text = font.render(seconds, True, WHITE)
text_rect = text.get_rect(center=(WINDOW_SIZE[0] // 2, 100))
screen.blit(text, text_rect)
# 更新显示
pygame.display.update()
```
这个代码通过 Pygame 库实现了一个简单的光立方,可以根据当前时间的秒数,显示对应的数字。在代码中,数字使用二进制字符串来表示,每个数字对应一个 4x3 的矩阵,其中 1 表示需要显示的部分,0 表示不需要显示的部分。绘制立方体的每个面时,根据数字字符串中的值来决定是否需要绘制该面。同时,代码还使用 Pygame 的文本渲染功能,在窗口中心绘制当前秒数的文本。
阅读全文
相关推荐
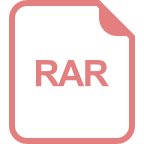
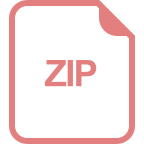
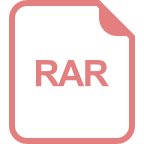
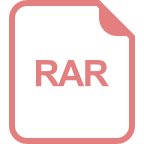
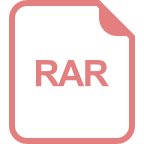
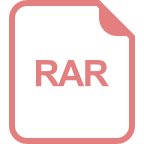
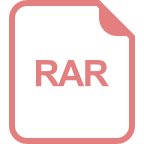
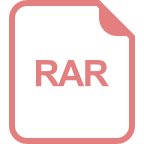
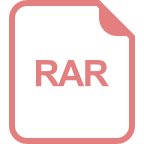
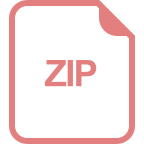
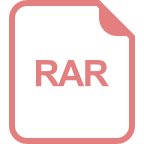
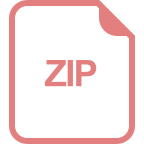
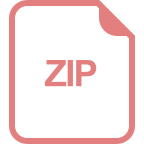
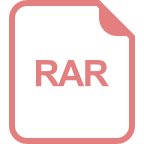
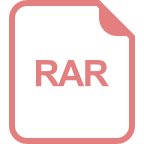
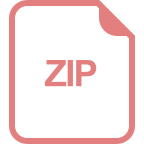
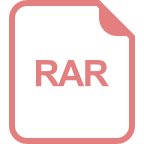